Mastering Docker: A Comprehensive Guide to Containerization and Deployment
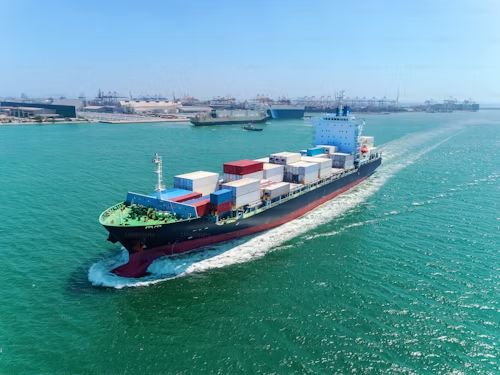
Mastering Docker: A Comprehensive Guide to Containerization and Deployment
Table of Contents (TOC):
Docker Architecture and Components
- 3.1 Docker Engine
- 3.2 Docker Images
- 3.3 Docker Containers
- 3.4 Docker Registries
- 3.5 Docker Volumes
Docker and Kubernetes: Introduction to Container Orchestration
-
- 17.1 Docker CLI Reference
- 17.2 Docker Command Cheatsheet
- 17.3 Additional Resources
Introduction to Docker
Docker has rapidly become one of the most popular and essential tools in the world of software development, DevOps, and cloud computing. In this chapter, we will explore Docker in detail, including what it is, why it's so useful, how it compares to virtual machines, and key concepts that underpin Docker’s containerization technology.
1.1 What is Docker?
At its core, Docker is an open-source platform that enables developers to build, ship, and run applications in isolated environments known as containers. A container is a lightweight, stand-alone, executable package that includes everything needed to run a piece of software: the code, runtime, libraries, system tools, and settings.
Docker was introduced in 2013 and has since revolutionized how developers deploy and manage applications. It enables developers to create consistent development environments that can be reproduced anywhere, from a local laptop to a cloud server.
How Docker Works
To better understand Docker, let’s break it down into a few key components:
Docker Engine: This is the runtime that runs and manages Docker containers. It consists of a server (the Docker daemon), a REST API, and a command-line interface (CLI).
Docker Containers: These are the isolated, executable packages. Containers allow you to run applications in a lightweight environment without worrying about inconsistencies between environments. They encapsulate everything the application needs to run.
Docker Images: An image is a blueprint for a container. It’s a snapshot of an application and all its dependencies. Images are typically stored in registries like Docker Hub or Docker Registry.
Docker Registry: A registry is a storage and distribution system for Docker images. Docker Hub is the most commonly used public registry, but organizations can also use private registries.
Simple Docker Example
Let’s say you want to run a simple web application, like a Node.js app. With Docker, you don’t need to worry about installing Node.js, npm, or any dependencies on your machine. You can simply pull a prebuilt image from Docker Hub and run it as a container.
Here’s a basic example:
- Pull an Image:
docker pull node
This pulls the official Node.js image from Docker Hub.
- Run the Container:
docker run -it --rm node
This starts a container based on the Node.js image and opens an interactive terminal session inside the container. The --rm
flag ensures that the container is removed once it's stopped.
Docker ensures that the container has everything the Node.js application needs to run, regardless of your local environment’s configuration.
1.2 Why Use Docker?
Docker provides a plethora of benefits that make it incredibly valuable in modern software development and operations.
1.2.1 Consistency Across Environments
One of the biggest challenges in development is ensuring that an application works the same way across different environments. This problem arises because every developer, test machine, staging environment, and production environment might have different configurations, versions of libraries, and operating systems.
With Docker, you can create an identical environment everywhere. A Docker container ensures that the application runs the same way, whether it’s running on a developer's laptop, a testing server, or in the cloud.
For example, let’s say you develop a Python application that works fine on your local machine but breaks on the production server. This inconsistency is often due to differences in system libraries, Python versions, or even OS configurations. With Docker, you package your app into a container that includes all dependencies, ensuring that it runs the same way everywhere.
1.2.2 Faster Development Cycles
Docker helps speed up the development process by making it easier to set up and manage environments. Developers can quickly spin up containers that replicate complex systems, allowing for faster testing, debugging, and iteration.
In traditional setups, developers need to manually install dependencies, which can be error-prone and time-consuming. With Docker, they can use Docker Compose to define multi-container setups in a single YAML file, drastically reducing the time spent on environment setup.
Example: A typical web application might require a database, a caching service, and a backend application. With Docker Compose, you can set all of these services up in one command.
version: '3'
services:
web:
image: my-web-app
ports:
- "5000:5000"
db:
image: postgres
environment:
POSTGRES_PASSWORD: example
Running docker-compose up
will bring up both the web app and the PostgreSQL database in their respective containers.
1.2.3 Isolation and Security
Docker containers provide process-level isolation. This means that processes inside a container cannot interfere with processes outside it. Containers are isolated from each other and from the host system, reducing the risk of bugs or security vulnerabilities affecting other applications.
For example, if a security vulnerability is discovered in a web application, Docker allows you to update or patch the application without affecting other services running in different containers.
Additionally, Docker has built-in support for user namespaces and Linux Security Modules (LSM), adding another layer of security.
1.2.4 Portability
Because Docker containers package all the dependencies required to run an application, they are highly portable. You can take a Docker container and run it anywhere, from a developer's local machine to the cloud. As long as the target system supports Docker, the application will run.
This is especially useful in cloud environments. You can run Docker containers on popular cloud services like AWS ECS, Google Cloud Run, or Azure Kubernetes Service.
1.2.5 Efficient Resource Usage
Docker containers are more lightweight than traditional virtual machines (VMs). Since containers share the host operating system's kernel, they use far less memory and storage than VMs, which require their own OS.
This makes Docker ideal for running many applications on a single machine. For example, a developer can run dozens of containers on their laptop without worrying about resource constraints.
1.3 Understanding Containerization
Containerization refers to the practice of packaging an application and its dependencies into a container that can be easily deployed and run anywhere.
How Containers Work
A container is similar to a virtual machine, but instead of virtualizing an entire operating system, it shares the host system’s OS kernel. This allows containers to be lightweight and fast. A container has everything it needs to run an application:
- The Application: The code that performs the required functionality.
- The Dependencies: Libraries, runtime environments, and frameworks needed for the application to run.
- System Tools and Settings: Configuration files, environment variables, and other system settings.
Containers do not include an operating system, but rather leverage the OS kernel of the host machine. This makes containers faster and less resource-intensive compared to virtual machines.
Docker vs. Traditional Virtualization
A Virtual Machine (VM) is a full emulation of a computer system. VMs run a complete operating system with its own kernel, which makes them resource-intensive. In contrast, Docker containers share the host’s kernel and only package the application and its dependencies, making them much lighter in terms of resource usage.
- VMs: Virtual machines are slower to start up because they need to load an entire OS. They also require more memory and disk space.
- Containers: Containers start almost instantly because they share the host system’s kernel and only include the application and its dependencies. Containers are much smaller in size, making them ideal for running multiple instances of an application on the same hardware.
1.4 Docker vs Virtual Machines
Let’s go into more detail on how Docker compares with virtual machines and why Docker’s containerization technology is often preferred over traditional virtualization.
1.4.1 Resource Efficiency
One of the most significant differences between Docker containers and virtual machines is the efficiency with which they use system resources. Since containers share the host OS kernel and do not require a full OS to be installed for each application, they use much less CPU and memory than virtual machines.
In contrast, VMs run an entirely separate OS, which means that each VM requires its own operating system, which consumes more resources. This overhead can become problematic when you want to run a large number of applications or microservices.
1.4.2 Performance
Containers outperform virtual machines in terms of speed. Since containers share the host OS kernel, they can start and stop in seconds, whereas VMs require more time because they need to boot up their own operating system.
This makes containers ideal for use cases where rapid scaling and fast start-up times are important, such as in microservices architectures or CI/CD pipelines.
1.4.3 Portability
While VMs can be moved from one host to another, they often require adjustments based on the underlying hypervisor or system configurations. Docker containers, on the other hand, are highly portable. As long as Docker is available on the host system, you can move containers between different machines without worrying about compatibility issues.
For example, a containerized application that runs on a developer’s laptop can be easily deployed to a testing environment, staging server, or production system without modification.
1.4.4 Security
Both Docker containers and virtual machines provide isolation, but in different ways. A virtual machine’s isolation is at the hardware level, meaning that a security
breach in one VM generally does not affect other VMs. Docker containers, on the other hand, provide isolation at the operating system level. While containers are isolated from one another, they share the same OS kernel, which can sometimes introduce security concerns.
That said, Docker has many built-in security features to prevent unauthorized access and limit damage if a container is compromised, such as container user namespaces and seccomp profiles.
1.4.5 Use Cases
- Virtual Machines: VMs are ideal for running applications that require full OS environments, such as legacy applications or systems that need to run different operating systems.
- Docker Containers: Docker is best suited for microservices, web apps, development environments, and applications that need to run in isolated, reproducible environments.
1.5 Key Docker Concepts
Before diving into Docker usage, it’s important to understand a few key concepts that form the foundation of the platform. These include Docker images, containers, Dockerfiles, volumes, and networks.
1.5.1 Docker Images
A Docker image is a snapshot of an application and its environment. It contains the application code, libraries, and dependencies, along with a file system structure. Docker images are the blueprints for creating Docker containers.
You can create your own Docker images or use pre-built images from the Docker Hub. For example, you can create an image for a Node.js application by creating a Dockerfile
, which is a script that contains the instructions for building the image.
Here’s an example of a simple Dockerfile
for a Node.js application:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 8080
CMD ["node", "app.js"]
This Dockerfile
tells Docker to use the official node
image as the base, copy the app's package.json
files, install dependencies, and run the app.
1.5.2 Containers
A Docker container is a running instance of a Docker image. While the image is the blueprint, the container is the actual running application. You can start, stop, and delete containers as needed.
To run a container based on an image, you use the docker run
command:
docker run -d -p 8080:8080 my-node-app
This starts the my-node-app
container in detached mode and maps port 8080 from the container to port 8080 on the host machine.
1.5.3 Volumes
Volumes in Docker are used to persist data generated by and used by containers. Since containers are ephemeral, meaning they are deleted when stopped, using volumes ensures that important data can be stored outside of the container and accessed later.
To create a volume, you can use the following command:
docker volume create my-volume
Then, mount the volume in a container like so:
docker run -v my-volume:/app/data my-node-app
This mounts the my-volume
volume to the /app/data
directory inside the container.
1.5.4 Docker Networks
Docker allows containers to communicate with each other through Docker networks. Docker provides several networking modes, such as bridge, host, and overlay networks, which allow you to control how containers connect to each other and the outside world.
To create a custom network:
docker network create my-network
And to run a container on a specific network:
docker run --network my-network my-node-app
This concludes the Introduction to Docker chapter. We have covered the basics of Docker, why it’s useful, the concept of containerization, and how Docker compares to traditional virtual machines. We've also introduced key concepts such as Docker images, containers, volumes, and networks. These foundational ideas will be essential as we dive deeper into Docker’s more advanced features in the following chapters.
Getting Started with Docker
Docker has revolutionized the way developers build, ship, and run applications by enabling them to package software into standardized units known as containers. These containers are portable, lightweight, and easy to deploy across different environments, making Docker an indispensable tool in modern software development and deployment pipelines.
In this chapter, we will cover the essential steps for getting started with Docker, including how to install Docker, verify your installation, use basic Docker commands, and run your first Docker container.
2.1 Installing Docker
2.1.1 Prerequisites
Before you install Docker, it's important to ensure that your system meets the necessary prerequisites. Docker supports various platforms, including Linux, macOS, and Windows.
- Linux: Docker supports most Linux distributions, such as Ubuntu, CentOS, Fedora, and Debian.
- macOS: Docker Desktop is available for macOS versions 10.14 or newer.
- Windows: Docker Desktop is also available for Windows 10 (Pro, Enterprise, or Education editions) and newer versions.
2.1.2 Installing Docker on Linux
Docker provides installation instructions specific to different Linux distributions. Here’s how to install Docker on Ubuntu, one of the most popular Linux distributions.
Step 1: Update the package list
First, ensure your system is up to date by running the following command:
sudo apt-get update
Step 2: Install dependencies
Docker requires some additional software packages to be installed first. Run the following command to install them:
sudo apt-get install apt-transport-https ca-certificates curl software-properties-common
Step 3: Add Docker’s official GPG key
Next, add Docker’s official GPG key so that your system can verify the authenticity of the Docker packages you will install:
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo apt-key add -
Step 4: Add Docker repository
Add the Docker APT repository to your system’s software sources:
sudo add-apt-repository "deb [arch=amd64] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable"
Step 5: Install Docker Engine
Now, install Docker by running:
sudo apt-get update
sudo apt-get install docker-ce
Step 6: Verify Docker installation
After installation is complete, verify that Docker is installed correctly by running:
sudo docker --version
This should display the Docker version installed, confirming the installation was successful.
2.1.3 Installing Docker on macOS
Installing Docker on macOS is straightforward. Here’s how you can do it:
Step 1: Download Docker Desktop
Go to the Docker website and download Docker Desktop for macOS.
Step 2: Install Docker Desktop
After the download is complete, open the .dmg
file and drag the Docker icon to the Applications folder.
Step 3: Run Docker
Once Docker is installed, open the "Applications" folder and launch Docker. The Docker icon will appear in the menu bar, indicating that the Docker daemon is running.
Step 4: Verify Docker installation
To confirm that Docker is installed correctly, open a terminal and run:
docker --version
This should display the Docker version installed on your system.
2.1.4 Installing Docker on Windows
Step 1: Download Docker Desktop for Windows
Visit the Docker website and download the Docker Desktop installer for Windows.
Step 2: Install Docker Desktop
Once the installer is downloaded, run the executable and follow the installation wizard. You may be prompted to enable the WSL 2 (Windows Subsystem for Linux) feature if you are using Windows 10. Docker Desktop uses WSL 2 for its Linux container support on Windows.
Step 3: Start Docker
Once the installation is complete, Docker Desktop should start automatically. If not, you can open it from the Start menu.
Step 4: Verify Docker installation
Open a terminal or Command Prompt and run:
docker --version
This should display the Docker version installed.
2.2 Verifying the Installation
After Docker is installed, you can verify that everything is working correctly by running a simple Docker command that checks the status of the Docker service.
2.2.1 Check Docker Version
The first thing you should do is check which version of Docker you have installed. This helps verify that Docker is properly installed on your system:
docker --version
This will return something like:
Docker version 20.10.5, build 55c4c88
If you see an error message instead, it might indicate that the Docker daemon isn’t running or that Docker wasn’t installed correctly.
2.2.2 Check Docker Daemon Status
To check if Docker is running on your system, use the following command:
On Linux:
sudo systemctl status docker
If Docker is running, you should see something like this:
● docker.service - Docker Application Container Engine
Loaded: loaded (/lib/systemd/system/docker.service; enabled; vendor preset: enabled)
Active: active (running) since Mon 2024-01-01 10:00:00 UTC; 2h 30min ago
If it’s not running, you can start Docker by using:
sudo systemctl start docker
On macOS and Windows:
Docker Desktop should manage the Docker daemon automatically. The Docker icon in the system tray or menu bar indicates whether Docker is running.
2.2.3 Running a Simple Test Container
Once Docker is up and running, it’s a good idea to test it by running a simple container. The official Docker image hello-world
is perfect for this:
docker run hello-world
This command tells Docker to download the hello-world
image from Docker Hub (if it's not already on your system), run it in a container, and display a message confirming that Docker is installed correctly.
You should see output similar to this:
Hello from Docker!
This message shows that your installation appears to be working correctly.
If you see this message, Docker is installed and working correctly.
2.3 Docker Command Line Basics
Now that Docker is installed and verified, let's explore some essential Docker commands that you’ll use on a daily basis.
2.3.1 Docker Help Command
The docker
command has a built-in help system. To list all available commands, use:
docker --help
To get help for a specific command, like docker run
, you can run:
docker run --help
This will display detailed information about how to use the docker run
command, including options and arguments.
2.3.2 Docker Images
Docker images are the blueprints from which containers are created. To list all the images on your system, run:
docker images
This will display output like:
REPOSITORY TAG IMAGE ID CREATED SIZE
hello-world latest 94e3f5d46c8a 2 months ago 13.3MB
ubuntu 20.04 47b19964fb50 4 weeks ago 64.2MB
You can remove an image using:
docker rmi <image_id>
2.3.3 Docker Containers
Containers are running instances of Docker images. You can list all containers (including stopped ones) using:
docker ps -a
This will display output like:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
12345abcde hello-world "/hello" 2 hours ago Exited (0) 2s hilarious_cori
To remove a container, use:
docker rm <container_id>
2.3.4 Running Containers
To run a container, use the docker run
command:
docker run <image_name>
For example, to run an Ubuntu container, you can type:
docker run -it ubuntu
This command starts a container in interactive mode (-it
) and opens a shell inside it. To exit the shell and stop the container, simply type exit
.
2.3.5 Stopping and Restarting Containers
To stop a running container, use the docker stop
command:
docker stop <container_id>
To restart a container, use:
docker start <container_id>
2.3.6 Docker Logs
To view the logs of a running or stopped container, use:
docker logs <container_id>
This is useful for debugging purposes.
2.4 Your First Docker Container
Now that you're familiar with some basic commands, let’s walk through running your first Docker container and exploring its features.
2.4.1 Running a Simple Web Server in a Container
In this example, we'll run a lightweight web server inside a Docker container using the nginx
image,
which is a popular web server.
- Pull the Nginx image:
docker pull nginx
This will download the Nginx image from Docker Hub if you don’t already have it locally.
- Run the container:
docker run -d -p 8080:80 --name my_nginx nginx
Here’s what this command does:
-d
: Run the container in detached mode (in the background).-p 8080:80
: Map port 8080 on your local machine to port 80 inside the container.--name my_nginx
: Assign the container a name (my_nginx
).nginx
: The image we’re using to run the container.
- Access the Web Server:
Now, open a browser and navigate to http://localhost:8080
. You should see the default Nginx welcome page.
- Stop the Container:
To stop the container, run:
docker stop my_nginx
- Remove the Container:
To remove the container when you're done, run:
docker rm my_nginx
2.4.2 Running a Custom Application in Docker
Now let’s run a custom application inside Docker. Suppose you have a Python script that you want to run in a container.
- Create a Python Script:
Create a file named app.py
with the following code:
print("Hello, Docker!")
- Create a Dockerfile:
Create a Dockerfile
that will define the environment for the Python application. Here’s a simple example:
# Use the official Python image
FROM python:3.8-slim
# Set the working directory inside the container
WORKDIR /app
# Copy the Python script into the container
COPY app.py .
# Run the script
CMD ["python", "app.py"]
- Build the Docker Image:
From the directory containing the Dockerfile
and app.py
, run:
docker build -t my-python-app .
- Run the Application:
Once the image is built, run the container:
docker run my-python-app
You should see the output:
Hello, Docker!
- Clean Up:
To stop and remove the container, use:
docker ps -a
docker rm <container_id>
You’ve now created a Docker container that runs a simple Python application.
Conclusion
In this chapter, you’ve learned how to install Docker, verify your installation, and run your first Docker container. You’ve also familiarized yourself with some basic Docker commands like docker ps
, docker run
, and docker logs
. Finally, you ran a simple web server and a custom Python application in Docker containers.
In the next chapters, we’ll dive deeper into Docker’s features, including creating custom images, working with Docker Compose, and deploying multi-container applications.
Docker Architecture and Components
Docker has revolutionized how developers, operations teams, and DevOps professionals build, ship, and run applications. At the heart of this transformation lies Docker's powerful architecture, which is designed for simplicity, scalability, and flexibility. Understanding Docker’s architecture and its various components is critical for anyone who wishes to effectively use Docker in a production environment or as part of a development pipeline.
In this chapter, we will explore the core components that make up Docker, each of which plays a crucial role in how Docker works. We will look at the Docker Engine, Docker Images, Docker Containers, Docker Registries, and Docker Volumes in detail, offering a comprehensive understanding of each concept, along with practical examples to reinforce the concepts.
3.1 Docker Engine
The Docker Engine is the core component of Docker. It is a client-server application that allows you to build, ship, and run Docker containers. It is composed of several different components that work together to enable containerization, and understanding how it works is essential for grasping Docker’s overall functionality.
3.1.1 Overview of Docker Engine Architecture
The Docker Engine consists of three primary components:
- Docker Daemon (
dockerd
): The Docker Daemon is responsible for managing Docker containers, images, networks, and volumes. It listens for requests from the Docker CLI (or other interfaces) and processes them. It handles tasks such as building containers, managing images, and starting or stopping containers. - Docker CLI (
docker
): The Docker Command Line Interface (CLI) allows users to interact with the Docker Daemon. The CLI is a command-line tool that sends requests to the Docker Daemon to perform operations on containers, images, and other Docker resources. - REST API: The Docker Daemon exposes a REST API that can be used by clients (such as the Docker CLI, Docker SDKs, or custom applications) to interact with the Docker Engine programmatically.
3.1.2 Docker Daemon
The Docker Daemon (dockerd
) is the background service that runs on a Docker host. It manages containers and images, and it can communicate with other Docker Daemons if you have a multi-host setup (using Docker Swarm or Kubernetes, for example).
The Docker Daemon listens on a Unix socket or a network port and is responsible for handling all the container management tasks such as:
- Building Docker Images: The Daemon builds images based on Dockerfiles, processes Docker builds, and pushes images to registries.
- Running Containers: The Daemon manages running containers, starting them when requested and stopping them when instructed.
- Managing Networks: Docker containers often need to communicate with each other. The Daemon manages network settings and configuration for containers, whether they’re part of a Docker network or exposed externally via port mappings.
- Managing Volumes: The Docker Daemon also handles persistent data for containers through Docker Volumes, ensuring that data is stored outside the container lifecycle.
3.1.3 Docker CLI
The Docker CLI (docker
) is a powerful command-line tool that interacts with the Docker Daemon. By issuing commands through the CLI, users can:
- Build Docker images (
docker build
) - List running containers (
docker ps
) - Run containers (
docker run
) - Push and pull images from Docker registries (
docker push
,docker pull
) - Create and manage networks (
docker network
) - Manage volumes (
docker volume
)
A simple example of using the Docker CLI to run a container:
docker run -d -p 80:80 --name web-server nginx
This command runs a container using the nginx
image, maps port 80 from the container to port 80 on the host, and names the container web-server
.
3.1.4 Docker REST API
The Docker Daemon exposes a REST API for external clients to interact with Docker programmatically. This API can be used to create containers, manage images, or handle container logs, all without using the CLI. For example, you can write Python, Go, or Java applications that interact with Docker through this API.
3.2 Docker Images
Docker Images are the building blocks of Docker containers. They are lightweight, standalone, and executable software packages that include everything needed to run a piece of software—code, runtime, libraries, environment variables, and configuration files.
3.2.1 What is a Docker Image?
A Docker image is a template used to create containers. It is an immutable, read-only file system that contains all the dependencies and instructions to run an application. Docker images are built from Dockerfiles, which define the steps to create an image, such as installing dependencies and copying files.
3.2.2 Dockerfile
A Dockerfile is a script that contains a series of instructions on how to build a Docker image. Each line in the Dockerfile creates a new layer in the image, which is cached for efficiency.
A simple example of a Dockerfile to create a Node.js application image:
# Use the official Node.js image as a base
FROM node:14
# Set the working directory inside the container
WORKDIR /app
# Copy package.json and install dependencies
COPY package.json .
RUN npm install
# Copy the rest of the application files
COPY . .
# Expose the application port
EXPOSE 3000
# Start the application
CMD ["npm", "start"]
The FROM
directive indicates the base image to use (in this case, Node.js), while RUN
executes commands like installing dependencies. The CMD
directive specifies the command to run when the container starts.
3.2.3 Layers in Docker Images
Docker images are built up from layers. Each layer represents an instruction in the Dockerfile. Layers can be reused across different images, which helps optimize storage and improve performance. For example, if multiple images use the same base image (FROM node:14
), the Docker engine will reuse the base image layer, saving space and time.
3.2.4 Building Docker Images
Once the Dockerfile is written, it can be used to build an image using the docker build
command:
docker build -t my-node-app .
This command tells Docker to build an image from the Dockerfile in the current directory (denoted by the .
) and tag it with the name my-node-app
.
3.2.5 Docker Image Registry
Once built, Docker images can be shared via Docker registries (such as Docker Hub or a private registry). Docker Hub is the default public registry where images can be pushed and pulled. By tagging an image with a registry URL, you can push it to a registry:
docker tag my-node-app username/my-node-app
docker push username/my-node-app
This allows teams to easily share and distribute Docker images.
3.3 Docker Containers
While Docker images are read-only templates, Docker containers are the instances created from images. Containers are the execution environments for your applications, and they are isolated from each other and from the host system.
3.3.1 What is a Docker Container?
A Docker container is a runtime instance of a Docker image. Containers are lightweight, fast, and isolated environments that contain everything necessary to run an application. They run on top of the Docker Engine and share the host system's kernel, but they are isolated from each other and the host system through namespaces and cgroups.
3.3.2 How Containers Work
When you run a Docker container using the docker run
command, Docker creates a container from the specified image, allocates a unique filesystem for it, and then starts the application inside it. The container runs in isolation, meaning that it has its own filesystem, networking, and processes. However, containers can share resources with the host system or other containers if needed.
A typical docker run
command:
docker run -d -p 8080:80 --name web-app my-node-app
This command:
- Runs a container in the background (
-d
) - Maps port 8080 on the host to port 80 on the container
- Names the container
web-app
- Uses the
my-node-app
image to create the container
3.3.3 Container Lifecycle
Containers go through several stages in their lifecycle:
- Create: A container is created from an image but not yet running.
- Start: The container begins execution and is running.
- Stop: The container is stopped gracefully.
- Restart: The container is restarted after being stopped.
- Remove: The container is deleted from the system.
You can view all the containers on your system with the following command:
docker ps -a
3.3.4 Container Isolation
Docker containers use Linux namespaces to achieve isolation. These namespaces provide a way to isolate processes, file systems, networks, and other resources. The following namespaces are key to Docker’s container isolation:
- PID namespace: Isolates process IDs (PID) so that processes in one container do not affect processes in another.
- Network namespace: Isolates networking, allowing each container to have its own IP address, ports, and network interfaces.
- Mount namespace: Isolates filesystem mounts, ensuring containers have their own filesystem layers.
- User namespace: Isolates user and group IDs to improve security.
- UTS namespace: Allows containers to have their own hostname.
3.3.5 Container Networking
Docker containers can communicate with each other using Docker's networking capabilities. Docker supports several networking modes:
- Bridge Mode: The default
networking mode. Containers on the same bridge network can communicate with each other using their container names.
- Host Mode: Containers share the host’s network stack, which can improve network performance.
- Overlay Mode: Used in Docker Swarm to connect containers running on different Docker hosts.
- None Mode: Containers have no network access.
3.4 Docker Registries
A Docker registry is a storage and distribution system for Docker images. Docker Hub is the default registry, but organizations can also set up private registries to store images securely.
3.4.1 Public vs. Private Registries
- Public Registries: Docker Hub is the most well-known public registry, where users can find and share images. Most popular official images are hosted here, including the official Nginx, Redis, and MySQL images.
- Private Registries: Organizations can host their own private registries for security reasons, ensuring that only authorized users have access to certain images.
3.4.2 Pulling and Pushing Images
You can pull images from a registry using the docker pull
command:
docker pull nginx
To push images to a registry, you first tag the image with the registry’s URL:
docker tag my-node-app myregistry.com/my-node-app
docker push myregistry.com/my-node-app
3.4.3 Docker Registry Architecture
A Docker registry consists of:
- Repositories: Groups of images that share a name. Each repository can have multiple versions of an image (tags).
- Tags: Tags are used to differentiate different versions of the same image. For example,
nginx:latest
ornginx:1.19.6
. - Layers: Docker images are built from layers, and these layers are stored in the registry. When an image is pulled, Docker only needs to pull the layers that are missing from the local system.
3.5 Docker Volumes
Docker Volumes are used to persist data outside of the container’s filesystem. Since containers are ephemeral by nature, any data stored inside a container will be lost when the container is removed. Volumes provide a way to store data persistently, ensuring it remains available even if the container is deleted or recreated.
3.5.1 What is a Docker Volume?
A Docker volume is a directory that exists outside of a container’s filesystem but is accessible to it. Volumes are typically stored in /var/lib/docker/volumes/
on the host system. Volumes are useful for storing database data, logs, configuration files, or any other data that needs to persist beyond a container’s lifecycle.
3.5.2 Creating and Using Volumes
You can create a volume using the following command:
docker volume create my-volume
To mount a volume inside a container:
docker run -d -v my-volume:/data my-image
In this case, the volume my-volume
is mounted to the /data
directory inside the container.
3.5.3 Volume Drivers
Docker supports various volume drivers that allow you to use different storage backends (e.g., local disks, cloud storage). You can specify the driver using the -driver
flag when creating a volume.
Conclusion
In this chapter, we’ve covered the fundamental components of Docker’s architecture, including the Docker Engine, Docker Images, Docker Containers, Docker Registries, and Docker Volumes. Understanding these components and how they interact with each other is key to effectively using Docker for developing and deploying applications. In the next chapter, we will dive into more advanced Docker topics, such as Docker Compose, Docker Swarm, and Docker networking, to further enhance your containerization skills.
Working with Docker Images
Docker has revolutionized the way developers build, ship, and run applications by providing a lightweight and consistent environment across different systems. One of the key elements in Docker's ecosystem is the concept of Docker Images. In this chapter, we will explore Docker Images in depth, covering everything from what they are, how to build custom ones, manage them, and optimize their size and performance. By the end of this chapter, you will have a solid understanding of how to work effectively with Docker Images in your development workflows.
4.1 What is a Docker Image?
A Docker image is essentially a blueprint for creating Docker containers. It is a packaged and immutable snapshot of an application and its environment, including everything needed to run the application, such as the operating system, software dependencies, application code, and configuration files.
Layers and the Union File System
Docker images are constructed in layers. Each layer represents a change made to the image, such as adding a file, installing a package, or setting an environment variable. These layers are built on top of a base image, which could be a minimal operating system like Alpine Linux or a more comprehensive image such as ubuntu
or node
.
Docker uses a Union File System (UFS) to combine these layers into a single, cohesive image. Each layer is read-only, and Docker uses a copy-on-write mechanism for the container to modify the image during runtime, allowing for efficient storage and transfer of images.
To visualize this, imagine an image consisting of three layers:
- Base layer: A minimal operating system (Ubuntu).
- Layer 2: The installation of Python.
- Layer 3: Your application code.
When you run a container based on this image, Docker creates a writable layer on top of these layers, where any changes you make inside the container (e.g., creating files or installing packages) will be stored.
Immutable and Portable
The key property of Docker images is that they are immutable. Once an image is built, it cannot be changed. Any modification to an image requires creating a new image with a new layer. This immutability makes Docker images highly portable, as they can be shared across different systems without worrying about inconsistencies in dependencies or configuration.
Docker Image Format
Docker images follow a specific format, where each image consists of the following components:
- Metadata: Contains basic information like the image’s name, tag, and a description of its contents.
- Filesystem Layers: These are the actual filesystem changes that define the image. They consist of files and directories created or modified during the build process.
- Configuration: Specifies how the container should run when the image is executed. This includes environment variables, default commands, entry points, and other configuration options.
- Layered History: A history of all layers in the image that allows Docker to rebuild or cache intermediate steps during image builds.
4.2 Building Custom Docker Images
While Docker provides many pre-built images in the Docker Hub (the central registry for Docker images), there are times when you need to build a custom image tailored to your specific needs. This could involve installing specific versions of software, configuring environment variables, or adding files that are necessary for your application to run.
The Dockerfile
The process of building a custom Docker image typically starts with a Dockerfile, a plain-text file that contains a series of instructions for Docker to follow in order to build an image. The Dockerfile defines the base image, installs dependencies, copies application code, and specifies the entry point for the container.
Here’s an example of a simple Dockerfile for building a Python-based web application using Flask:
# Step 1: Choose a base image
FROM python:3.8-slim
# Step 2: Set the working directory inside the container
WORKDIR /app
# Step 3: Copy the local application code to the container
COPY . /app
# Step 4: Install any dependencies specified in the requirements.txt file
RUN pip install --no-cache-dir -r requirements.txt
# Step 5: Expose the port that the application will use
EXPOSE 5000
# Step 6: Specify the command to run when the container starts
CMD ["python", "app.py"]
Let’s break down each instruction in the Dockerfile:
- FROM python:3.8-slim: This is the base image. In this case, it's a lightweight version of Python 3.8.
- WORKDIR /app: This sets the working directory for the following instructions to
/app
. - COPY . /app: This copies all files from the current directory on your host machine (where the Dockerfile resides) to the
/app
directory inside the container. - RUN pip install -r requirements.txt: This installs Python dependencies using
pip
. TheRUN
command executes the given command inside the image during the build. - EXPOSE 5000: This informs Docker that the container will listen on port 5000 (the default port for Flask).
- CMD ["python", "app.py"]: This sets the default command that will run when the container starts.
Building the Docker Image
Once you have your Dockerfile ready, you can build the image using the docker build
command. Here’s how you do it:
docker build -t my-flask-app .
In this command:
-t my-flask-app
: This specifies the name of the image you’re building (you can also tag the image with a version, e.g.,my-flask-app:v1
)..
: This tells Docker to look for the Dockerfile in the current directory.
Docker will then execute the instructions in the Dockerfile, layer by layer, and create a new image.
Using Build Context
The build context refers to the files and directories that are available to Docker during the build process. By default, Docker uses the contents of the current directory (denoted by .
) as the build context. However, you can specify a different directory if needed.
Handling Cache During Build
Docker caches each layer of the image during the build process. This allows Docker to reuse layers that haven’t changed, speeding up future builds. If you modify a layer that was previously cached, Docker will rebuild that layer and all subsequent layers.
You can control caching behavior using the --no-cache
flag, which forces Docker to rebuild all layers from scratch:
docker build --no-cache -t my-flask-app .
4.3 Managing Docker Images
Once you have created Docker images, managing them effectively is crucial to ensure that your system remains organized and efficient. Docker provides several commands for listing, inspecting, tagging, and deleting images.
Listing Docker Images
To see all the images currently available on your system, you can use the docker images
or docker image ls
command:
docker images
This will show a table with columns for:
- Repository: The name of the image.
- Tag: The version or tag of the image (e.g.,
latest
orv1
). - Image ID: A unique identifier for the image.
- Created: The timestamp when the image was created.
- Size: The size of the image.
Inspecting Docker Images
You can inspect an image to get detailed information about it using the docker inspect
command:
docker inspect my-flask-app
This will return detailed metadata in JSON format, such as:
- The base image it was built from.
- The environment variables and configuration used in the image.
- The command used to run the container.
Tagging Docker Images
Docker images are often tagged to specify different versions. For example, you can tag an image with v1.0
and then later tag it as latest
. You can create or modify tags using the docker tag
command:
docker tag my-flask-app my-flask-app:v1.0
docker tag my-flask-app my-flask-app:latest
Deleting Docker Images
Over time, you may accumulate many images that are no longer needed. To delete an image, use the docker rmi
command followed by the image name or ID:
docker rmi my-flask-app
If the image is being used by a running container, Docker will not allow the image to be removed unless you force it with the -f
flag:
docker rmi -f my-flask-app
You can also remove unused images (dangling images) with the following command:
docker image prune
This command will remove all images that are not tagged and are not associated with any container.
4.4 Optimizing Docker Images
While Docker images are designed to be lightweight, they can sometimes become large and bloated due to unnecessary files or inefficient build steps. Optimizing Docker images is essential for reducing their size, improving build performance, and reducing the attack surface of your containers.
Use Minimal Base Images
One of the most effective ways to optimize Docker images is to start with a minimal base image. For example, instead of using ubuntu
, consider using alpine
, a small, security-focused Linux distribution.
FROM python:3.8-alpine
Alpine images are typically much smaller than their Debian or Ubuntu counterparts, making them an excellent choice for many applications.
Minimize the Number of Layers
Each instruction in a Dockerfile (e.g., RUN
, COPY
, `ADD
`) creates a new layer in the Docker image. Having too many layers can increase the size of the image and the time it takes to build.
To minimize layers, combine related commands into a single RUN
statement:
RUN apt-get update && apt-get install -y curl && apt-get clean
This combines the package installation and cleaning commands into a single layer, rather than creating separate layers for each command.
Clean Up After Installation
Sometimes, when you install software dependencies, additional files or caches are created that are not needed in the final image. For instance, package managers like apt
or npm
may leave behind cache files that unnecessarily increase the size of the image.
To avoid this, clean up any unnecessary files after installation:
RUN apt-get update && apt-get install -y curl && rm -rf /var/lib/apt/lists/*
This command ensures that the package manager cache is removed after installation, reducing the image size.
Use Multi-Stage Builds
Multi-stage builds allow you to separate the build process into different stages, each using a different base image. This helps to reduce the final size of the image by only including the necessary components in the final stage.
Here’s an example of a multi-stage Dockerfile for building a Go application:
# First stage: Build the application
FROM golang:1.16-alpine AS builder
WORKDIR /app
COPY . .
RUN go build -o myapp .
# Second stage: Create the final image
FROM alpine:latest
WORKDIR /app
COPY --from=builder /app/myapp .
ENTRYPOINT ["./myapp"]
In this example:
- The first stage uses the
golang:1.16-alpine
image to build the application. - The second stage uses a minimal
alpine
image, and it only copies the compiled binary from the first stage, avoiding the inclusion of the Go build tools and source code in the final image.
Reduce the Number of Dependencies
Only include the dependencies that are absolutely necessary for your application to run. If you are working with a language that has many libraries or frameworks, consider using tools to generate a minimal set of dependencies. For example:
- For Python, use
pip freeze
to lock your dependencies in arequirements.txt
file and avoid unnecessary packages. - For Node.js, use
npm ci
instead ofnpm install
to install exactly what is defined inpackage-lock.json
.
Conclusion
In this chapter, we explored Docker images from the ground up. We discussed what Docker images are, how to build custom images using a Dockerfile, how to manage them, and various techniques for optimizing them. By understanding the underlying structure and best practices for managing and optimizing Docker images, you can create efficient and lightweight images that can be shared, deployed, and scaled in a seamless manner across different environments. Docker images are a powerful tool for modern development workflows, and mastering them is key to creating effective and performant containerized applications.
Managing Containers
Containers have become a fundamental part of modern software development and deployment. Docker, as the leading containerization platform, provides a comprehensive set of tools for creating, managing, and orchestrating containers. In this chapter, we'll explore how to effectively run Docker containers, manage their lifecycle, configure networking, and work with container logs. These skills are essential for any developer or system administrator working with Docker in production environments.
5.1 Running Docker Containers
Running containers in Docker is a straightforward process, but understanding the full range of options available for managing containers will help you create more efficient and secure environments.
5.1.1 Docker run
Command Overview
The most basic command to run a container is docker run
. It combines several actions into one: pulling the image from a registry (if it isn't already available locally), creating a container from that image, and starting the container. Here's a basic example:
docker run hello-world
The above command does the following:
- It pulls the
hello-world
image from Docker Hub (if not already present). - It creates a new container from the image.
- It starts the container and runs its default command.
Key Flags with docker run
-d
: Run the container in detached mode (in the background).docker run -d nginx
The
nginx
container will run in the background.-p
: Expose container ports to the host system. For example, to map the container's port 80 to the host's port 8080, you can use:docker run -d -p 8080:80 nginx
--name
: Assign a specific name to the container. By default, Docker assigns a random name to containers, but you can specify your own:docker run -d --name my_nginx -p 8080:80 nginx
-e
: Set environment variables inside the container:docker run -d -e MY_VAR=value nginx
-v
: Mount a volume from the host into the container to persist data or share files:docker run -d -v /host/path:/container/path nginx
This maps a directory on the host to a directory inside the container.
5.1.2 Running Containers with Specific Images
In most cases, you’ll be working with a variety of images, either from Docker Hub or from your own private registries. Docker provides an easy way to specify which image to use when running a container:
docker run -d --name my_mysql -e MYSQL_ROOT_PASSWORD=root -p 3306:3306 mysql:latest
This command:
- Starts a MySQL container with the root password set to
root
. - Maps port 3306 of the container to port 3306 on the host.
- Runs the container using the
mysql:latest
image from Docker Hub.
5.1.3 Running Containers Interactively
Sometimes you need to run a container interactively to debug or perform administrative tasks inside the container. You can use the -it
flag to attach an interactive terminal session to a container:
docker run -it ubuntu bash
This command will:
- Start a new container based on the
ubuntu
image. - Run the
bash
shell inside the container. - Attach your terminal to the running container, allowing you to interact with it.
5.2 Managing Container Lifecycle
Managing the lifecycle of Docker containers involves starting, stopping, restarting, and removing containers as necessary. Docker provides various commands to handle these operations.
5.2.1 Starting and Stopping Containers
Once a container is created (either by running a new container or by starting an existing one), you can manage its lifecycle using the following commands:
Starting a stopped container:
docker start <container_name_or_id>
Stopping a running container:
docker stop <container_name_or_id>
By default,
docker stop
sends aSIGTERM
signal to gracefully stop the container. If the container doesn't stop within 10 seconds, Docker will send aSIGKILL
signal.Stopping a container immediately (forcefully):
docker kill <container_name_or_id>
This command forces the container to stop without any grace period.
5.2.2 Restarting Containers
Sometimes you may want to restart a container after making configuration changes or troubleshooting. Docker provides a simple way to restart containers:
docker restart <container_name_or_id>
This command will stop and then immediately start the container.
5.2.3 Removing Containers
When you no longer need a container, you can remove it from the system. To remove a stopped container:
docker rm <container_name_or_id>
You can also remove a container while it is running by first stopping it:
docker stop <container_name_or_id> && docker rm <container_name_or_id>
If you want to remove a container and its associated volumes, use the -v
flag:
docker rm -v <container_name_or_id>
5.2.4 Viewing Container Status
To see all the containers running on your system, you can use the docker ps
command. By default, it only shows running containers. To list all containers (including stopped ones), use the -a
flag:
docker ps -a
This will display information such as the container ID, name, status, ports, and the image used.
To see logs from a container:
docker logs <container_name_or_id>
This provides the logs generated by the container’s main process.
5.3 Container Networking
In Docker, containers communicate with each other using networking. Docker provides several networking modes to accommodate various use cases.
5.3.1 Default Networking Mode: Bridge
By default, Docker containers use the "bridge" network mode. In this mode, Docker creates a virtual bridge interface on the host system, and each container gets its own private IP address on this bridge network. Containers can communicate with each other over this network, but they are isolated from the host system unless port mappings are configured.
You can specify the bridge network explicitly when starting a container:
docker run -d --name my_nginx --network bridge nginx
5.3.2 Host Networking
In the host network mode, a container shares the host's network namespace. This means the container will have the same IP address as the host and can use any of the host's network interfaces.
docker run -d --name my_nginx --network host nginx
Use this network mode when the container needs to access specific host resources or if you need high-performance networking.
5.3.3 Custom Networks
You can create custom Docker networks to enable containers to communicate with each other in isolated groups. For instance:
docker network create my_network
After creating the network, you can run containers on this network:
docker run -d --name my_nginx --network my_network nginx
You can also connect existing containers to a custom network:
docker network connect my_network my_nginx
To disconnect a container from a network:
docker network disconnect my_network my_nginx
5.3.4 Exposing Ports and Host-Container Communication
When running containers that need to interact with the outside world (e.g., web servers, databases), you must expose container ports to the host system using the -p
flag, as shown previously. This enables applications on the host to access services running inside the container.
For example, if you are running a web server inside a container and want to access it via the host’s port 8080, you would do:
docker run -d -p 8080:80 nginx
This maps the container’s internal port 80 to the host’s port 8080.
5.4 Working with Docker Logs
Logs are an essential part of understanding what’s happening inside a container. Docker makes it easy to view logs for both running and stopped containers.
5.4.1 Viewing Logs with docker logs
You can use the docker logs
command to retrieve logs from a container. By default, Docker stores logs for each container in its own logging driver. The most common logging driver is the default "json-file" driver, which stores logs in JSON format.
docker logs <container_name_or_id>
This command will display the standard output and standard error (stdout/stderr) generated by the container’s main process.
Follow Logs in Real-Time
If you want to tail the logs and view them in real-time (similar to the tail -f
command), use the -f
flag:
docker logs -f <container_name_or_id>
5.4.2 Viewing Specific Log Output
You can view only stdout or stderr logs by using the --stdout
and --stderr
flags (available in specific log drivers).
To view logs from the last N lines:
docker logs --tail N <container_name_or_id>
``
`
For example, to view the last 100 lines:
```bash
docker logs --tail 100 <container_name_or_id>
5.4.3 Using Log Drivers for Custom Logging Solutions
Docker allows you to configure different logging drivers that control how logs are handled. The default logging driver is json-file
, but you can change it to others like syslog
, journald
, fluentd
, or even a remote log server.
To configure the logging driver for a container, use the --log-driver
option:
docker run -d --log-driver=syslog nginx
This sends the container’s logs to the syslog service instead of the default file-based logging.
Conclusion
Managing Docker containers is a fundamental skill that every developer and systems administrator should master. Understanding how to run containers, manage their lifecycle, configure networking, and access logs will help you create and maintain efficient, secure containerized applications. This chapter covered the most commonly used commands and practices for managing Docker containers in various environments. Mastery of these concepts will empower you to troubleshoot, optimize, and scale your containerized applications effectively.
Docker Compose: Simplifying Multi-Container Applications
In modern software development, applications have become more complex, often requiring multiple services to work together seamlessly. This can include databases, web servers, caching systems, message brokers, and other components that must be configured and managed together. Docker, a tool that allows developers to package applications and their dependencies into containers, has made managing these services much simpler. However, when dealing with applications that require multiple containers, managing them individually can become cumbersome.
This is where Docker Compose comes into play. Docker Compose is a tool designed to define and manage multi-container Docker applications. With Docker Compose, you can define the configuration for all the services in your application using a simple YAML file, and Docker Compose will handle the orchestration of these services. In this chapter, we will explore Docker Compose in detail, walking through its functionality, how to use it, and how it simplifies the management of complex, multi-container applications.
6.1 Introduction to Docker Compose
Docker Compose was developed to address the complexity of managing multi-container applications. Instead of managing each container individually, Docker Compose allows you to define your entire application stack in a single file, making it easy to run, scale, and maintain. The application stack could be composed of different services such as:
- Web servers (e.g., Nginx, Apache)
- Databases (e.g., MySQL, PostgreSQL)
- Application services (e.g., Python Flask, Node.js)
- Caching systems (e.g., Redis, Memcached)
- Message queues (e.g., RabbitMQ, Kafka)
Without Docker Compose, managing these services individually can be time-consuming and error-prone, especially when they need to interact with each other. Docker Compose solves this problem by enabling the use of a single YAML file (docker-compose.yml
) to configure multiple services.
Key Features of Docker Compose:
- Single configuration file: Define all services, networks, and volumes in one YAML file.
- Easy orchestration: Start, stop, and manage the lifecycle of multi-container applications with simple commands.
- Service dependencies: Docker Compose allows you to specify service dependencies, so they are started in the correct order.
- Service scaling: Scale services horizontally by increasing the number of containers.
- Environment configuration: Easily manage environment variables and configuration options for each service.
How Docker Compose Works:
Docker Compose works by defining a set of containers as services in a docker-compose.yml
file. Each service is typically a single container that runs a specific part of your application. You can also define networks and volumes within the Compose file to allow containers to communicate with each other and share data. Once the file is set up, you can start all of the services with a single command, and Docker Compose will take care of creating the containers, networks, and volumes.
In the following sections, we'll delve deeper into how Docker Compose is set up, how to write the configuration file, and how to manage multi-container applications.
6.2 Creating a docker-compose.yml File
The docker-compose.yml
file is the core of Docker Compose. It’s where you define the services that make up your application, along with networks and volumes. This file uses YAML (Yet Another Markup Language), which is easy to read and write.
Basic Structure of a docker-compose.yml File
The general structure of a docker-compose.yml
file consists of the following key sections:
- version: Defines the version of the Compose file format.
- services: Defines the different services (containers) that make up your application.
- networks: Specifies custom networks that your services will use.
- volumes: Defines persistent storage volumes that can be shared among containers.
Here’s an example of a simple docker-compose.yml
file that defines a web server (using Nginx) and a database (using MySQL):
version: "3.9" # Specify Docker Compose file format version
services:
web:
image: nginx:latest # Use the latest version of the Nginx image
ports:
- "80:80" # Map port 80 on the host to port 80 on the container
networks:
- mynetwork # Connect the web service to the 'mynetwork'
db:
image: mysql:5.7 # Use the MySQL 5.7 image
environment:
MYSQL_ROOT_PASSWORD: rootpassword # Set root password for MySQL
MYSQL_DATABASE: mydb # Create a database named 'mydb'
volumes:
- db_data:/var/lib/mysql # Mount a volume for persistent database data
networks:
- mynetwork # Connect the db service to the same network as the web service
networks:
mynetwork: # Define a custom network for the services to communicate
volumes:
db_data: # Define a named volume to persist database data across restarts
Explanation of the docker-compose.yml
Example:
- version: The version specifies the syntax and features supported by Docker Compose. The version "3.9" is commonly used in production environments. Each new version introduces more advanced features, so it's important to use the correct version for your needs.
- services: In this example, we define two services—
web
anddb
:- web: The Nginx service uses the
nginx:latest
Docker image. We expose port 80 from the container to port 80 on the host machine, which allows us to access the web server through the host’s IP address or localhost. - db: The MySQL service uses the
mysql:5.7
image, and we set the environment variables for the root password and database name. We also define a volume (db_data
) to store the MySQL data persistently, even if the container is stopped or removed.
- web: The Nginx service uses the
- networks: Both services are connected to a custom network called
mynetwork
. This allows them to communicate with each other using container names as hostnames (e.g., theweb
container can connect to thedb
container usingdb
as the hostname). - volumes: The
db_data
volume ensures that MySQL data is persisted across container restarts. If you remove the MySQL container, the data will still be available in the volume.
Running the Application:
Once the docker-compose.yml
file is created, you can run the application using the following commands:
# Start the application (will start both the web and db services)
docker-compose up
# Run in detached mode (background)
docker-compose up -d
# Stop the application
docker-compose down
These commands allow you to start and stop your entire application with a single command, which is one of the main benefits of using Docker Compose.
6.3 Managing Multi-Container Applications
Once the docker-compose.yml
file is in place, Docker Compose simplifies the management of multi-container applications. It provides commands to start, stop, and manage the lifecycle of your containers. Let’s explore some common operations in more detail.
Starting the Application
To start all the services defined in your docker-compose.yml
file, use the following command:
docker-compose up
This command does the following:
- Downloads any necessary Docker images that are not already on your system.
- Creates and starts the containers for each service defined in the Compose file.
- Sets up the networks and volumes as defined.
To start the services in the background, use the -d
(detached) flag:
docker-compose up -d
Stopping the Application
To stop all running services, use the following command:
docker-compose down
This stops and removes all the containers associated with the application, along with the default network. However, any volumes that are defined in the docker-compose.yml
file are preserved by default to avoid data loss.
Restarting the Application
To restart the services, you can either stop and then start them again using docker-compose down
and docker-compose up
, or you can use the following command to restart the services in place:
docker-compose restart
This command only restarts the containers without removing them. It can be useful if you need to refresh the state of your containers.
Viewing Logs
Docker Compose provides an easy way to view the logs of all running services. To view the logs for all services:
docker-compose logs
If you want to view the logs for a specific service, such as the web
service, you can specify the service name:
docker-compose logs web
You can also use the -f
flag to follow the logs in real-time:
docker-compose logs -f
Executing Commands in Running Containers
You can execute commands inside a running container using the docker-compose exec
command. For example, to open a bash shell inside the web
container, you can run:
docker-compose exec web bash
This is useful for debugging, inspecting files, or running commands directly within a container.
Scaling Services
One of the powerful features of Docker Compose is the ability to scale services. Scaling allows you to run multiple instances (replicas) of a service. For example, you might want to run multiple web server containers behind a load balancer to handle more traffic.
To scale a service, use the --scale
option:
docker-compose up --scale web=3
This command will start three instances of the web
service
. The number after the equals sign specifies how many containers to run for that service. If you have a load balancer or reverse proxy in place (e.g., Nginx or HAProxy), it can distribute incoming requests among the multiple instances.
Removing Unused Containers and Volumes
When you run docker-compose down
, it will remove the containers but leave the volumes intact. If you want to remove the volumes as well (to free up disk space or reset the data), you can run:
docker-compose down -v
This will remove both the containers and the associated volumes.
6.4 Scaling and Extending Applications
In real-world applications, the need to scale and extend your services is crucial. Docker Compose makes it easy to scale services horizontally (adding more containers) and extend applications by adding new services or features.
Scaling Services Horizontally
In the previous section, we saw how to scale a service by running multiple containers for a single service. This is particularly useful for web servers, API servers, or any stateless services that can handle multiple replicas.
For example, if you want to scale the web service to handle more traffic, you can run multiple containers:
docker-compose up --scale web=5
This will create five instances of the web
service. You can also scale other services in the same way.
When scaling services that need to be load-balanced, ensure that you have a load balancer (like Nginx) to distribute incoming traffic to the containers. The docker-compose.yml
file might look like this:
version: "3.9"
services:
web:
image: nginx:latest
ports:
- "80:80"
deploy:
replicas: 3 # Scale to 3 instances of web service
loadbalancer:
image: nginx:latest
ports:
- "80:80"
links:
- web
volumes:
- ./nginx.conf:/etc/nginx/nginx.conf
In this setup, we are using Nginx as a reverse proxy to route traffic to the multiple web server instances.
Extending Applications with New Services
In a microservices-based architecture, applications often consist of multiple services. Docker Compose allows you to easily extend your applications by adding new services. Let’s say you want to add a caching layer to your application using Redis. You can simply add the Redis service to your docker-compose.yml
file:
services:
web:
image: nginx:latest
ports:
- "80:80"
db:
image: mysql:5.7
environment:
MYSQL_ROOT_PASSWORD: rootpassword
volumes:
- db_data:/var/lib/mysql
redis:
image: redis:latest
networks:
- mynetwork
In this example, we’ve added a Redis service to the application, which is connected to the same network (mynetwork
) as the web and database services. You can then update your application code to use Redis as a caching layer.
Conclusion
Docker Compose simplifies the management of multi-container applications by allowing you to define your application stack in a single YAML file. By using Docker Compose, you can easily start, stop, and scale your application services with simple commands. It also provides a convenient way to manage networks and persistent volumes.
Whether you are working with a single container or multiple interconnected services, Docker Compose helps streamline the deployment and orchestration process, making it an indispensable tool for developers working in containerized environments. By mastering Docker Compose, you can greatly improve the efficiency and maintainability of your application infrastructure.
Orchestrating with Docker Swarm
7.1 What is Docker Swarm?
Docker Swarm is Docker's native clustering and orchestration solution that allows you to deploy and manage a cluster of Docker containers as a single, unified system. It transforms multiple Docker hosts (machines) into a single logical unit, referred to as a Swarm, allowing you to run containerized applications in a scalable and highly available manner.
Swarm provides the following key features:
Service Discovery: In a Swarm cluster, each container can be discovered using a built-in DNS service. Services can communicate with each other by their service name, making it easier to scale applications and manage containers in a distributed system.
High Availability: Docker Swarm ensures that the desired state of your application is maintained. If a container goes down, Swarm automatically redeploys it to another available node in the cluster, ensuring continuous operation and high availability.
Load Balancing: Swarm has built-in load balancing capabilities, which distribute incoming traffic to the containers across the cluster. This ensures that traffic is balanced effectively among all the available nodes.
Multi-Node Clustering: Swarm allows you to create a cluster with multiple nodes (both manager and worker nodes). This helps you scale your applications horizontally, with a manager node overseeing the overall management and worker nodes executing the actual workload.
Declarative Service Model: Swarm uses a declarative approach to manage services. You define the desired state (e.g., the number of replicas, network configuration, resource limits), and Swarm will ensure the actual state matches the desired state.
Rolling Updates: Docker Swarm allows you to roll out updates to services without downtime. You can perform rolling updates, where containers are updated one at a time or in batches, so that the application remains available during the update process.
Security: Docker Swarm automatically encrypts the traffic between nodes in the cluster. It also supports role-based access control (RBAC) to manage who can perform specific actions in the swarm.
Components of Docker Swarm
Docker Swarm consists of two main components:
Manager Nodes: These nodes are responsible for the orchestration and management of the Swarm cluster. They store the cluster state and perform tasks such as scheduling services, maintaining the desired state, and managing the overall health of the cluster.
Worker Nodes: These nodes execute the tasks assigned to them by the manager nodes. Worker nodes run the containers and report their status to the manager nodes.
Swarm uses an internal Raft-based consensus algorithm to ensure that all manager nodes in the cluster have the same view of the cluster state, providing fault tolerance and consistency.
7.2 Setting Up Docker Swarm
Setting up Docker Swarm is a straightforward process that involves initializing a Swarm on a single node and then adding additional nodes to the cluster. In this section, we will walk through the process of setting up Docker Swarm from scratch.
Step 1: Install Docker on All Nodes
Before setting up Docker Swarm, you need to install Docker on all the machines (virtual or physical) that will be part of the cluster. You can follow the Docker installation guide for the platform-specific installation steps.
For example, to install Docker on Ubuntu:
# Update the package index
sudo apt-get update
# Install dependencies
sudo apt-get install apt-transport-https ca-certificates curl software-properties-common
# Add Docker's official GPG key
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo apt-key add -
# Set up the stable Docker repository
sudo add-apt-repository "deb [arch=amd64] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable"
# Update the package index again
sudo apt-get update
# Install Docker CE (Community Edition)
sudo apt-get install docker-ce
Repeat this process on all the nodes you want to include in your Swarm cluster.
Step 2: Initialize the Docker Swarm
Once Docker is installed on all nodes, you can initialize the Swarm on the first node, which will be the "manager" node.
On the manager node, run the following command to initialize the Swarm:
docker swarm init --advertise-addr <MANAGER-IP>
<MANAGER-IP>
is the IP address of the manager node that other nodes in the cluster will use to join the Swarm.
The command will output a token that worker nodes will use to join the Swarm cluster. The output will look like this:
To add a worker to this swarm, run the following command:
docker swarm join --token <WORKER-TOKEN> <MANAGER-IP>:2377
Step 3: Join Worker Nodes to the Swarm
On each worker node, run the docker swarm join
command using the token provided in the previous step. For example:
docker swarm join --token <WORKER-TOKEN> <MANAGER-IP>:2377
After running this command, the worker nodes will join the Swarm cluster, and the manager node will now be aware of all the nodes in the cluster.
Step 4: Verify the Cluster
Once you’ve added all worker nodes to the Swarm, you can verify that the cluster is set up correctly. On the manager node, run:
docker node ls
This command will show a list of all nodes in the Swarm cluster, their roles (manager or worker), and their current status.
Example output:
ID HOSTNAME STATUS AVAILABILITY MANAGER STATUS
x67ghw0rfljwgldbf0tm1d3n4 manager-node Ready Active Leader
q9hf3f4t6s6mj7ny13n5l9gv0 worker-node-1 Ready Active
h80rf6ss7t77r0v92zpk0byga worker-node-2 Ready Active
The STATUS
column shows the current status of the nodes, and the MANAGER STATUS
column indicates if the node is a manager and its role in the Swarm (e.g., Leader
or Reachable
).
Step 5: Inspect the Swarm
You can also inspect the details of the Swarm to view information like the node’s availability, service information, and more:
docker info
This will show the current Docker daemon information, including the Swarm state.
Step 6: (Optional) Add More Manager Nodes
If you need high availability for managing the Swarm, you can add more manager nodes. This is useful in production environments to ensure that if one manager fails, others can take over. You can promote an existing worker node to a manager using the following command:
docker node promote <NODE-ID>
To demote a manager node back to a worker, you can use:
docker node demote <NODE-ID>
7.3 Managing Services and Tasks
Once the Swarm is set up, you can start deploying applications as services. A service in Docker Swarm represents a set of identical containers running on the cluster. The containers in a service are known as tasks.
Creating a Service
To create a service in Docker Swarm, use the docker service create
command. For example, to create a simple NGINX service with three replicas, run:
docker service create --name nginx-service --replicas 3 -p 80:80 nginx
--name nginx-service
: Specifies the name of the service.--replicas 3
: Specifies the number of replicas (tasks) you want to run for the service.-p 80:80
: Maps port 80 of the container to port 80 on the host machine.nginx
: The image to use for the service (NGINX in this case).
Docker Swarm will automatically schedule these three replicas across the available nodes, ensuring that the desired number of tasks are running at any given time.
Listing Services
To see all the services running in the Swarm cluster, use the following command:
docker service ls
This will display the services running on the Swarm, their replicas, and their status.
Example output:
ID NAME MODE REPLICAS IMAGE
bdf5xxq7jkp7 nginx-service replicated 3/3 nginx
Inspecting a Service
You can inspect a service to see detailed information, such as the number of tasks, the image being used, and the ports exposed:
docker service inspect nginx-service
This command will return a JSON output with detailed information about the service, including the current state of all tasks associated with the service.
Scaling a Service
You can scale a service by adjusting the number of replicas. For example, to scale the NGINX service to 5 replicas, use:
docker service scale nginx-service=5
Swarm will automatically adjust the number of tasks to match the desired state, and it will distribute the new tasks across the available nodes in the cluster.
Updating a Service
You can update the configuration of a service, such as changing the image or adding environment variables. For example, to update the NGINX service to use a newer version of the image, you would run:
docker service update --image nginx:latest nginx-service
This will cause Docker Swarm to gradually replace the old containers with the new version, ensuring zero downtime.
Removing a Service
To remove a
service from the Swarm, run the following command:
docker service rm nginx-service
This will stop and remove all tasks related to the service and clean up the resources.
Viewing Tasks in a Service
You can inspect the tasks running for a service using:
docker service ps nginx-service
This will show you the individual tasks (containers) running for the service, their status, and the node where they are running.
7.4 Scaling and Load Balancing in Swarm
Docker Swarm has built-in features for scaling services and load balancing traffic across the containers in the cluster. Let's dive into how these work.
Scaling Services
Scaling services allows you to handle varying loads by adjusting the number of replicas. Swarm will ensure that the desired number of tasks are always running and distribute them across the available worker nodes.
For example, to scale the nginx-service
to 10 replicas:
docker service scale nginx-service=10
Swarm will automatically deploy 7 more tasks (containers) and spread them across the worker nodes. If a node runs out of resources, Swarm will try to place the tasks on other nodes.
Load Balancing
Docker Swarm has built-in load balancing, which automatically distributes incoming traffic to the containers in a service. Swarm does this through the routing of traffic to containers based on DNS round-robin.
When you create a service and expose a port (e.g., -p 80:80
), Swarm will load balance the traffic to the different replicas of that service. It ensures that traffic is distributed across the containers, and if one container goes down, Swarm will route the traffic to the remaining containers.
To test the load balancing, you can run a simple HTTP service (like the NGINX example above) and send traffic to the exposed port. If you have multiple replicas, the traffic will be distributed across all the containers, even as they are scaled up or down.
Rolling Updates with Load Balancing
Swarm ensures that there is no downtime when updating services. When you perform a rolling update, it updates containers one by one or in batches, making sure that the service remains available during the process.
For example, to update the nginx-service
to use the latest NGINX image, you can run:
docker service update --image nginx:latest nginx-service
Swarm will update the service in a way that ensures that only a fraction of the replicas are updated at any given time, allowing the rest of the replicas to handle incoming traffic while the update happens. This guarantees zero downtime and smooth transitions during the update process.
Conclusion
Docker Swarm offers a powerful and simple way to orchestrate and manage containerized applications in a distributed environment. Its ease of setup, automatic load balancing, service discovery, and high availability features make it a great choice for production-grade applications. By scaling services and taking advantage of rolling updates, Docker Swarm enables seamless application deployment and management.
Docker in Production: Best Practices
Docker has become the de facto standard for deploying and managing applications in containers. Containers provide isolation, consistency, and scalability, making them an ideal choice for production environments. However, deploying Docker in production requires careful planning and adherence to best practices to ensure your containers are secure, efficient, and easy to maintain. In this chapter, we will explore several key areas of Docker in production, including security considerations, performance optimization, continuous integration (CI) practices, and deployment strategies.
8.1 Security Considerations
Security is one of the most critical aspects when running Docker in production. While Docker offers a level of isolation between containers, it is essential to understand that containers are not immune to security threats. The attack surface in a Dockerized environment is substantial, and therefore, security should be a top priority.
8.1.1 Container Image Security
One of the first places to ensure security is by using secure container images. These images can be vulnerable if they contain outdated libraries, insecure configurations, or software bugs.
1. Use Trusted Base Images
Always start with trusted base images from reputable sources such as the official Docker Hub repositories or private, verified registries. For instance, the official nginx
or alpine
images are widely recognized for their security practices.
# Use official NGINX image as the base
FROM nginx:latest
When building custom images, avoid using latest
tags for base images, as this can result in unpredictable vulnerabilities over time. Instead, pin the image version to a specific tag or digest.
# Use a fixed version for the base image
FROM nginx:1.21.4
2. Scan Your Images for Vulnerabilities
Before deploying a container to production, it’s important to scan the image for vulnerabilities. There are several tools available for this, such as:
- Clair: A static analysis tool for detecting vulnerabilities in Docker images.
- Trivy: A simple and comprehensive vulnerability scanner.
- Docker Scan: Built into Docker CLI to scan images for vulnerabilities.
To use Docker Scan, simply run:
docker scan <image_name>
3. Minimize the Size of Your Images
Smaller images typically have fewer potential vulnerabilities because they contain fewer packages and dependencies. Use multi-stage builds in your Dockerfiles to create smaller production images by separating the build environment from the runtime environment.
# Stage 1: Build the application
FROM node:14 AS build
WORKDIR /app
COPY . .
RUN npm install
RUN npm run build
# Stage 2: Create a smaller runtime image
FROM nginx:alpine
COPY --from=build /app/dist /usr/share/nginx/html
By using the multi-stage build, only the necessary files and dependencies are copied to the final image, reducing its size and potential attack surface.
4. Avoid Running Containers as Root
Running containers with root privileges increases the attack surface. Always run containers with a non-root user, if possible. You can specify a user in the Dockerfile using the USER
directive.
# Add a non-root user
RUN adduser -D myuser
USER myuser
8.1.2 Container Runtime Security
The Docker daemon is responsible for managing containers, and if it's compromised, an attacker could gain control over all containers. Securing the Docker runtime is critical.
1. Limit Docker Daemon Privileges
Ensure that Docker is running with the least amount of privileges necessary. Avoid running Docker as root, and instead, manage Docker permissions through user groups or use tools like Docker's --user
flag to limit the user who can interact with Docker.
sudo usermod -aG docker <username>
2. Enable Docker Content Trust (DCT)
Docker Content Trust (DCT) ensures that the images pulled from the Docker registry are signed and verified. This helps prevent the use of tampered or malicious images.
You can enable Docker Content Trust by setting the DOCKER_CONTENT_TRUST
environment variable to 1
.
export DOCKER_CONTENT_TRUST=1
8.1.3 Network and Runtime Security
Networking and runtime security are vital for protecting the communication between containers, and between containers and external services.
1. Use Docker Networks
Containers in Docker can communicate with each other over networks. By default, Docker uses the bridge
network, but it’s best practice to create custom networks to isolate containers and provide more granular control.
docker network create --driver=bridge my_network
When defining services in a docker-compose.yml
file, you can specify the network to which the service belongs:
version: "3.7"
services:
app:
image: myapp
networks:
- my_network
networks:
my_network:
driver: bridge
2. Use Docker Secrets for Sensitive Data
For storing sensitive data like passwords, API keys, or certificates, Docker Secrets is a safer method than environment variables or configuration files. Secrets are encrypted and only available to containers that need them.
To create a secret:
echo "my_secret_password" | docker secret create db_password -
And in your service definition, you can access the secret:
services:
app:
image: myapp
secrets:
- db_password
secrets:
db_password:
external: true
3. Enforce Seccomp and AppArmor Profiles
Seccomp and AppArmor provide kernel-level security by restricting the system calls a container can make. By default, Docker applies a default Seccomp profile, but you can create a custom one to limit system calls even further.
docker run --security-opt seccomp=/path/to/custom-seccomp-profile.json myapp
8.1.4 Logging and Monitoring
Effective monitoring and logging help in detecting potential security incidents in real-time. Ensure that your containers are configured to log important events, and use a centralized logging solution like ELK Stack or Prometheus.
docker run -d --log-driver=syslog myapp
8.2 Optimizing Docker Performance
Performance optimization is essential to ensure that Docker containers run efficiently, without consuming unnecessary resources or suffering from bottlenecks. Docker provides various techniques and tools for fine-tuning container performance.
8.2.1 Image Optimization
1. Reduce the Number of Layers
Each command in a Dockerfile creates a new layer. Too many layers can increase the image size and complexity. You can reduce the number of layers by combining commands where possible.
# Before
RUN apt-get update
RUN apt-get install -y curl
# After
RUN apt-get update && apt-get install -y curl
2. Clean Up Unused Dependencies
If you're installing development dependencies during the build phase, ensure they’re removed after the build is complete.
RUN apt-get install -y build-essential && \
make myapp && \
apt-get remove --purge build-essential && \
apt-get autoremove && \
rm -rf /var/lib/apt/lists/*
3. Use Multi-stage Builds
As mentioned previously, multi-stage builds help separate build and runtime environments, ensuring that only essential files are included in the final image.
8.2.2 Resource Limiting
Docker allows you to limit the CPU and memory usage for each container. This is important to prevent a single container from consuming too many resources and affecting the overall system’s performance.
docker run --memory="512m" --cpus="1.0" myapp
In a production setting, use docker-compose.yml
to specify resource limits for services:
version: "3"
services:
app:
image: myapp
deploy:
resources:
limits:
cpus: '0.5'
memory: 512M
8.2.3 Docker Swarm and Orchestration
When scaling Docker containers in production, orchestration tools like Docker Swarm or Kubernetes help automate deployment, scaling, and management. They provide load balancing, service discovery, and fault tolerance, which significantly enhance performance.
- Docker Swarm: Docker’s native clustering and orchestration tool for managing multiple containers across multiple nodes.
- Kubernetes: A more robust container orchestration platform that supports Docker and other container runtimes.
8.2.4 Disk I/O Optimization
Disk I/O can become a bottleneck when containers write a lot of data to disk. To optimize disk I/O, consider the following:
- Use
tmpfs
for ephemeral storage if data doesn’t need to persist across restarts.
docker run --mount type=tmpfs,destination=/app/tmp myapp
- Mount external volumes when you need to persist data across container restarts.
docker run -v /host/path:/container/path myapp
8.3 Continuous Integration (CI) with Docker
Integrating Docker into your CI pipeline improves development velocity, consistency, and reliability. Docker ensures that the environment in which your code is built and tested is the same as in production, reducing the risk of deployment failures.
8.3.1 Setting Up a CI Pipeline
A common
practice in Docker CI pipelines is to build a Docker image, run tests in the container, and then push the image to a registry if the tests pass. This can be accomplished using CI tools like Jenkins, GitLab CI, CircleCI, or GitHub Actions.
Example using GitHub Actions:
name: Docker CI Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Build and push Docker image
run: |
docker build -t myapp:$GITHUB_SHA .
docker push myapp:$GITHUB_SHA
In this example, the CI pipeline automatically builds the Docker image and pushes it to a registry upon code changes to the main branch.
8.3.2 Running Tests in Docker Containers
You can run unit tests, integration tests, and even end-to-end tests inside Docker containers as part of the CI process. This ensures that the tests are run in an environment identical to production.
- name: Run Tests
run: |
docker run --rm myapp:test npm test
8.3.3 Automating Docker Image Builds and Deployments
You can automate Docker image builds and deployments by integrating your CI pipeline with cloud providers like AWS, Google Cloud, or Azure. This allows you to deploy your application to staging or production environments seamlessly once the image is built and tested.
8.4 Deploying to Production
Deploying Docker containers in production requires a well-defined strategy to ensure stability, scalability, and high availability. Several tools and techniques can help in orchestrating the deployment process.
8.4.1 Docker Compose for Development and Staging
Docker Compose is an excellent tool for managing multi-container applications during development and staging. It allows you to define your services in a docker-compose.yml
file and spin up your entire environment with a single command.
docker-compose up -d
8.4.2 Docker Swarm for Production Orchestration
For production environments, Docker Swarm provides a simple orchestration solution that supports scaling, service discovery, and automated load balancing across multiple nodes.
docker swarm init
docker stack deploy -c docker-compose.yml myapp
8.4.3 Kubernetes for Large-Scale Production
For larger-scale applications, Kubernetes provides a more robust orchestration solution with advanced features like auto-scaling, rolling updates, and service meshes.
kubectl apply -f myapp-deployment.yaml
8.4.4 Blue-Green Deployment
Blue-green deployment is a strategy where two environments (blue and green) are maintained. One environment is live (blue), and the other (green) is used for testing new releases. After testing, traffic is switched to the green environment.
docker run -d --name blue myapp:v1
docker run -d --name green myapp:v2
8.4.5 Rollback Strategy
Having a rollback strategy is crucial in case the new deployment fails. Both Docker Swarm and Kubernetes offer simple ways to rollback deployments.
docker service rollback myapp
kubectl rollout undo deployment/myapp
This concludes our expanded discussion of "Docker in Production: Best Practices." By following these best practices, you can ensure that your Dockerized applications are secure, efficient, and reliable in a production environment.
Docker and Kubernetes: Introduction to Container Orchestration
In the world of modern software development and deployment, containers have revolutionized the way applications are built, shipped, and run. Docker has become the de facto standard for containerization, providing developers with an easy-to-use platform to package applications and their dependencies into portable containers. However, while Docker handles individual containers, the management and orchestration of containers in large-scale, production environments require a more sophisticated approach.
This is where Kubernetes comes into play. Kubernetes is an open-source container orchestration platform that automates the deployment, scaling, and management of containerized applications. Together, Docker and Kubernetes form the foundation of modern DevOps practices, enabling teams to manage applications with greater ease and efficiency.
In this chapter, we will explore the differences between Kubernetes and Docker Swarm, demonstrate how Docker can be integrated with Kubernetes, discuss how containers are managed within a Kubernetes cluster, and delve into networking between Docker containers in Kubernetes environments.
9.1 Kubernetes vs Docker Swarm
Before diving deeper into Kubernetes and Docker integration, it’s important to understand the two most common orchestration tools used in conjunction with Docker: Kubernetes and Docker Swarm. Both of these tools are used to manage and orchestrate Docker containers, but they approach this task in different ways.
Overview of Docker Swarm
Docker Swarm is Docker's native clustering and orchestration solution. It allows developers to deploy and manage a cluster of Docker nodes as a single virtual host. Swarm simplifies the management of Docker containers across multiple machines, providing a way to scale applications, manage service discovery, load balancing, and more.
Key Features of Docker Swarm:
- Native Docker integration: Since Swarm is built into Docker, it is easy to set up and use, with minimal configuration required.
- Simplified setup: Docker Swarm offers a relatively easy setup for small to medium-scale applications, making it suitable for simpler use cases.
- Built-in load balancing: Docker Swarm automatically distributes requests across containers, providing basic load balancing features.
- Declarative service model: Swarm uses a declarative model to define the desired state of services, allowing it to automatically manage container scaling and fault tolerance.
However, as the scale of deployment grows, Docker Swarm can start to show limitations in areas such as flexibility, scalability, and advanced configuration. This is where Kubernetes shines.
Overview of Kubernetes
Kubernetes (often abbreviated as K8s) is a much more comprehensive container orchestration platform that goes beyond basic container management. Initially developed by Google, Kubernetes has become the industry standard for managing containerized applications at scale. Unlike Docker Swarm, Kubernetes provides extensive features for managing large and complex clusters, making it suitable for large enterprise environments.
Key Features of Kubernetes:
- High scalability: Kubernetes can scale applications easily, even to thousands of containers across multiple nodes.
- Advanced scheduling: Kubernetes uses sophisticated algorithms to schedule containers optimally across nodes based on resource usage, ensuring efficient utilization of infrastructure.
- Self-healing: If a container crashes, Kubernetes automatically restarts it or replaces it, providing high availability and minimizing downtime.
- Advanced networking and storage: Kubernetes has built-in support for complex networking and persistent storage, making it ideal for stateful applications.
- Rich ecosystem: Kubernetes integrates with a wide range of tools for monitoring, logging, CI/CD, and security, creating a full-featured ecosystem for modern application management.
Comparing Kubernetes and Docker Swarm
While both Kubernetes and Docker Swarm handle container orchestration, they differ significantly in terms of complexity, scalability, and feature set:
Feature | Docker Swarm | Kubernetes |
---|---|---|
Ease of Setup | Easy to set up; ideal for small clusters | More complex; requires configuration |
Scaling | Limited scalability | Handles large-scale deployments with ease |
Networking | Simple networking | Advanced networking with pod-to-pod communication |
Service Discovery | Built-in service discovery | More advanced service discovery with DNS and other features |
Fault Tolerance | Basic, limited to container restart | Advanced self-healing with replication and auto-scaling |
Ecosystem | Limited ecosystem | Rich ecosystem with integrations for CI/CD, monitoring, and more |
Community and Adoption | Popular but less widely adopted than K8s | The industry standard for large-scale container orchestration |
When to Use Docker Swarm
Docker Swarm is ideal for smaller projects or teams that need to quickly get up and running with a simple orchestration system. It’s a good choice if:
- You have a relatively small deployment with less complex requirements.
- You need a solution with minimal configuration.
- You are already heavily invested in Docker.
When to Use Kubernetes
Kubernetes is the go-to choice for large, complex, or mission-critical applications. It is best suited for:
- Large-scale deployments that require high availability and fault tolerance.
- Teams that need to manage complex distributed systems.
- Environments with microservices architectures or stateful applications.
- Organizations looking for a robust ecosystem of tools for CI/CD, monitoring, and security.
9.2 Integrating Docker with Kubernetes
One of the great things about Kubernetes is that it was designed to work with any container runtime that supports the Open Container Initiative (OCI) standards, including Docker. Although Kubernetes is often used with Docker, the integration between the two requires understanding a few key concepts: pods, containers, and Kubernetes nodes.
Key Concepts:
- Docker Containers: At the core of Docker is the container. A container is a lightweight, standalone, and executable software package that includes everything needed to run a piece of software, including the code, runtime, libraries, and system tools.
- Pods: In Kubernetes, containers are not managed individually but are grouped into pods. A pod is the smallest and simplest Kubernetes object that represents a set of containers that share the same network namespace, storage, and resources.
- Nodes: A node in Kubernetes represents a single machine in the cluster that runs one or more pods. A Kubernetes cluster consists of a set of nodes that work together to run containerized applications.
Setting Up Docker with Kubernetes
To get started with Docker and Kubernetes, we’ll assume that you have both Docker and Kubernetes installed. If you’re using a local environment for testing, you can use Minikube, which runs a single-node Kubernetes cluster on your local machine. For production-grade deployments, you would typically use cloud-based Kubernetes services such as Google Kubernetes Engine (GKE), Amazon Elastic Kubernetes Service (EKS), or Azure Kubernetes Service (AKS).
Step 1: Install Minikube and Docker
First, install Minikube and Docker on your local machine. Follow the installation instructions for your operating system from their respective official sites.
For example, on Ubuntu, you can install Minikube as follows:
curl -LO https://storage.googleapis.com/minikube/releases/latest/minikube-linux-amd64
sudo mv minikube-linux-amd64 /usr/local/bin/minikube
sudo chmod +x /usr/local/bin/minikube
Start your Minikube instance:
minikube start
Next, ensure Docker is installed and running. On Ubuntu, you can install Docker with:
sudo apt-get install docker.io
Make sure Docker is running:
sudo systemctl start docker
sudo systemctl enable docker
Step 2: Create a Docker Container
Next, let's build a simple Docker container that we can deploy on Kubernetes. We'll create a basic web application in Python using Flask.
First, create a directory for your project:
mkdir flask-app
cd flask-app
Create a Python file app.py
:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, Docker and Kubernetes!'
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0', port=5000)
Next, create a Dockerfile
to containerize the application:
FROM python:3.8-slim
# Set the working directory inside the container
WORKDIR /app
# Copy the app files to the container
COPY app.py .
# Install Flask
RUN pip install flask
# Expose the port that Flask is running on
EXPOSE 5000
# Run the application
CMD ["python", "app.py"]
Now build the Docker image:
docker build -t flask-app .
Test the image locally:
docker run -p 5000:5000 flask-app
You can open a browser and go to http://localhost:5000
to verify that the app is running.
Step 3: Create Kubernetes Deployment for Docker Container
Now that the Docker image is ready, we can create a Kubernetes deployment to run this container. First, create a Kubernetes configuration file deployment.yaml
:
apiVersion: apps/v1
kind: Deployment
metadata:
name: flask-deployment
spec:
replicas: 1
selector:
matchLabels:
app: flask
template:
metadata:
labels:
app: flask
spec:
containers:
- name: flask
image: flask-app
ports:
- containerPort: 5000
This YAML file defines a deployment for your Flask application. It specifies that Kubernetes should run one replica of the container and expose port 5000.
To apply the deployment to Kubernetes, use the following
command:
kubectl apply -f deployment.yaml
Once the deployment is created, you can check the status with:
kubectl get deployments
You can also check the running pods:
kubectl get pods
If everything is set up correctly, the container will be running inside your Kubernetes cluster.
9.3 Managing Containers in Kubernetes
Managing containers in Kubernetes involves working with multiple objects and controllers, such as Deployments, Services, Ingresses, and Namespaces. These components help you manage the lifecycle of containers, provide scaling and self-healing, and expose your application to external users.
Key Kubernetes Objects for Container Management
Pods
A Pod is the smallest deployable unit in Kubernetes and consists of one or more containers. Pods are the logical host for containers and provide a shared network, storage, and context for the containers within them.
Deployments
A Deployment manages the lifecycle of pods, ensuring that the desired number of pod replicas are running at all times. It automatically handles scaling, rolling updates, and rollbacks for your applications.
ReplicaSets
A ReplicaSet ensures that a specified number of identical pods are running at any given time. While deployments automatically create and manage ReplicaSets, you can manually create a ReplicaSet if needed.
Services
A Service is an abstraction that defines a set of pods and a policy by which to access them. Services are used for load balancing and service discovery, allowing communication between different parts of an application.
Example: Scaling with Kubernetes
To scale your application in Kubernetes, you simply need to update the replica count in the Deployment configuration. For example, to scale the Flask application to three replicas:
apiVersion: apps/v1
kind: Deployment
metadata:
name: flask-deployment
spec:
replicas: 3
selector:
matchLabels:
app: flask
template:
metadata:
labels:
app: flask
spec:
containers:
- name: flask
image: flask-app
ports:
- containerPort: 5000
To apply the changes:
kubectl apply -f deployment.yaml
Kubernetes will automatically create two more pods to match the desired state, ensuring high availability.
9.4 Kubernetes Networking with Docker
Networking is one of the more complex aspects of Kubernetes, but it is also one of the most powerful features. In Kubernetes, containers communicate with each other over the pod network. The network model in Kubernetes is designed to allow all containers in a pod to communicate with each other, and for pods on different nodes to communicate as well.
Kubernetes Network Model
In Kubernetes, every pod gets its own unique IP address. Containers within the same pod can communicate with each other using localhost
, while containers in different pods communicate via their respective pod IPs.
Service Discovery
Kubernetes uses services to enable discovery and load balancing between containers. Each service gets a stable DNS name that can be used by other containers to communicate with it. For example, if you define a service for your Flask application, other containers in the cluster can access it using the service name.
Here is an example of a simple Kubernetes service definition:
apiVersion: v1
kind: Service
metadata:
name: flask-service
spec:
selector:
app: flask
ports:
- protocol: TCP
port: 80
targetPort: 5000
This service will expose port 5000 of the Flask container on port 80 inside the cluster.
Conclusion
Docker and Kubernetes are powerful tools that, when used together, can significantly simplify the process of deploying, managing, and scaling containerized applications. While Docker handles individual containers, Kubernetes offers advanced features for orchestrating and managing containers across large, complex clusters. By understanding the differences between Docker Swarm and Kubernetes, and learning how to integrate Docker with Kubernetes, you can ensure that your applications are scalable, resilient, and easy to manage in a production environment.
In the next sections, we will continue to explore more advanced features and real-world scenarios where Docker and Kubernetes can work together to streamline your application lifecycle management.
Docker for Developers: A Practical Guide
In this chapter, we'll explore how Docker can revolutionize the way developers build, test, and deploy applications. We’ll start by discussing how to "Dockerize" applications, moving through Docker for development environments, unit testing inside Docker containers, and debugging applications running in containers. This guide will walk through practical examples, providing the necessary tools and best practices for integrating Docker into your development workflow.
10.1 Dockerizing Applications
Dockerizing an application refers to the process of packaging the application code, its dependencies, libraries, and environment into a container image, ensuring it can run consistently across different environments—whether it's a local machine, a staging server, or a cloud provider. Docker achieves this by using a lightweight virtualized environment known as a container, which encapsulates everything the application needs to run.
What is Dockerizing an Application?
When we say we're "Dockerizing" an application, we're essentially taking the app and putting it into a Docker container. This allows developers to ensure that the application will behave the same way on any machine, regardless of whether it's running locally, on a colleague’s system, or on a cloud-based server. This eliminates the "it works on my machine" problem.
For this purpose, Docker uses two primary concepts:
- Docker Image: A snapshot of the application and its dependencies.
- Docker Container: A running instance of the Docker image.
Steps to Dockerize an Application
Install Docker: Ensure Docker is installed on your local machine. You can download Docker Desktop for Windows or macOS, or Docker Engine for Linux.
Create a Dockerfile: A
Dockerfile
is a text file that contains a series of instructions on how to build a Docker image for your application. Here’s an exampleDockerfile
for a Python-based web app:
# Use an official Python runtime as the base image
FROM python:3.9-slim
# Set the working directory inside the container
WORKDIR /app
# Copy the current directory contents into the container
COPY . /app
# Install any needed dependencies
RUN pip install --no-cache-dir -r requirements.txt
# Expose the port the app runs on
EXPOSE 5000
# Define environment variable
ENV FLASK_APP=app.py
# Run the application
CMD ["flask", "run", "--host=0.0.0.0"]
Explanation:
FROM
: Specifies the base image (in this case, a slim version of Python 3.9).WORKDIR
: Defines the directory inside the container where the app will reside.COPY
: Copies the current working directory to the/app
directory in the container.RUN
: Executes commands, like installing dependencies, inside the container.EXPOSE
: Opens the port on which the app will run.CMD
: Specifies the command to run when the container starts (in this case, a Flask app).
- Build the Docker Image: After defining the
Dockerfile
, you build the image using thedocker build
command:
docker build -t my-python-app .
This will build a Docker image tagged my-python-app
based on the instructions in your Dockerfile
.
- Run the Docker Container: Now that the image is built, you can run a container from it:
docker run -p 5000:5000 my-python-app
This command tells Docker to map port 5000 on your local machine to port 5000 in the container (the port your app is running on).
Dockerizing Other Applications
The process of Dockerizing varies slightly depending on the type of application, but the principles remain the same. Here are a few brief examples:
- Node.js Application:
# Use Node.js as the base image
FROM node:16
# Set working directory
WORKDIR /app
# Copy application files
COPY . /app
# Install dependencies
RUN npm install
# Expose app port
EXPOSE 3000
# Start the application
CMD ["npm", "start"]
- Java Application (Spring Boot):
# Use OpenJDK base image
FROM openjdk:11-jre-slim
# Copy jar file from target directory
COPY target/myapp.jar /app/myapp.jar
# Expose application port
EXPOSE 8080
# Run the application
CMD ["java", "-jar", "/app/myapp.jar"]
10.2 Docker for Development Environments
Docker can be a game-changer when it comes to managing development environments. Often, developers face challenges with different versions of libraries, database configurations, or system dependencies. Docker can help by ensuring that every developer works in the same environment, regardless of the host machine.
Benefits of Using Docker for Development
- Consistency: Docker ensures that the development environment is consistent across all team members and environments (development, staging, production).
- Isolation: Docker containers run in isolation from one another, ensuring that dependencies don't conflict.
- Reproducibility: You can easily recreate an environment from a Docker image by simply pulling the image and running it.
Example: Docker Compose for Multi-container Environments
In modern development, applications often depend on multiple services: a database, caching service, queue, etc. Docker Compose is a tool for defining and running multi-container Docker applications. A single docker-compose.yml
file can define all of these services and their interconnections.
For instance, consider a web application that uses a Flask backend and a PostgreSQL database. Here’s how you could define the environment using Docker Compose:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
depends_on:
- db
db:
image: postgres:13
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
POSTGRES_DB: mydb
volumes:
- postgres_data:/var/lib/postgresql/data
volumes:
postgres_data:
Explanation:
web
: This section defines the Flask web application. Thebuild
directive tells Docker Compose to build the image from theDockerfile
in the current directory.db
: This defines the PostgreSQL database service. The environment variables specify the username, password, and database name.depends_on
: Ensures that the web service starts only after the database service is up and running.
To start the environment, run:
docker-compose up
Docker Compose will pull the necessary images (if they’re not already present), build the web
service, and start the containers.
10.3 Running Unit Tests with Docker
Running unit tests inside Docker containers ensures that tests are executed in an environment that mimics production, improving the reliability and consistency of your tests.
Why Run Unit Tests in Docker?
- Consistency: The tests will always run in the same environment, reducing variability caused by differing local environments.
- Speed: Running tests in isolated containers can speed up CI/CD pipelines.
- Isolation: Containers allow tests to run without interfering with the host environment or other tests.
Example: Running Unit Tests in a Python Application
Suppose you have a Python application with the following structure:
/app
/tests
test_example.py
app.py
requirements.txt
Dockerfile
You can run unit tests inside Docker by using a Dockerfile
like this:
# Use an official Python runtime
FROM python:3.9-slim
# Set working directory
WORKDIR /app
# Copy the application and test files
COPY . /app
# Install dependencies
RUN pip install --no-cache-dir -r requirements.txt
# Run unit tests
CMD ["pytest", "tests"]
This Dockerfile
installs the dependencies and runs tests using pytest
. To build and run the tests in a Docker container, use the following commands:
docker build -t my-python-app .
docker run my-python-app
If you're using a more complex application or multiple services (e.g., a backend service that relies on a database), you can extend this setup with Docker Compose to manage the dependencies for running tests. You could add a test service to your docker-compose.yml
file:
version: '3'
services:
web:
build: .
command: pytest tests
depends_on:
- db
db:
image: postgres:13
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
POSTGRES_DB: mydb
This will automatically run the tests when the container starts.
10.4 Debugging Applications Inside Containers
Debugging applications inside Docker containers can be challenging due to the isolation and lack of a direct interface with the running process. However, Docker provides several tools and techniques for debugging applications running inside containers.
Methods of Debugging Dockerized Applications
Attach to Running Containers: You can attach to a running container's console to interact with it in real time.
docker exec -it <container_name_or_id> /bin/bash
This command allows you to get a shell inside the container. Once inside, you can inspect logs, check processes, and run commands interactively.
Viewing Logs: Docker provides a built-in logging mechanism to capture stdout and stderr from running containers. To view logs, use the
docker logs
command:“`bash
docker logs
This is useful for inspecting output generated by the application running inside the container.
3. **Interactive Debugging with Debugger Tools**:
For more advanced debugging, especially for applications in languages like Python, Node.js, or Java, you can use debuggers that integrate with Docker.
For Python, for example, you could use `pdb` (Python Debugger) to debug your code inside the container. Modify your `Dockerfile` to include the `pdb` module and set breakpoints:
```python
import pdb
pdb.set_trace()
Then rebuild your container and run it as usual. When the breakpoint is hit, you can interactively debug inside the container's terminal.
Remote Debugging for Web Applications: For applications like Node.js, remote debugging can be enabled by adding the following to your Dockerfile:
EXPOSE 9229 CMD ["node", "--inspect=0.0.0.0:9229", "app.js"]
Then, you can attach to the application’s debugger using tools like Chrome DevTools or Visual Studio Code.
Docker Desktop GUI: Docker Desktop provides a graphical user interface for managing containers, viewing logs, inspecting resource usage, and even accessing shell sessions. While not as flexible as using the command line, it’s a handy tool for developers who prefer GUI-based workflows.
Conclusion
Dockerizing your applications, setting up consistent development environments, running tests inside containers, and debugging applications within the containerized environment are crucial practices for modern software development. Docker enables a level of portability and reliability that is difficult to achieve with traditional development setups. By integrating Docker into your workflow, you can improve collaboration, streamline development, and eliminate many of the common issues associated with environment inconsistencies and deployment headaches.
With Docker, developers can move from writing code on their local machine to running it in production without worrying about how the application will behave across different systems. As we’ve seen in this chapter, Docker can be leveraged not only for deployment but also for managing the development lifecycle, making it an indispensable tool for modern developers.
Deploying Docker Containers in the Cloud
As organizations increasingly adopt microservices architecture, containerization has become a vital part of the development and deployment process. Docker, one of the most widely used containerization tools, allows developers to build, package, and run applications in isolated environments known as containers. These containers encapsulate everything the application needs to run, including the application code, runtime, system tools, and libraries, making them portable and easy to deploy across various environments.
Cloud platforms such as AWS, Google Cloud Platform (GCP), and Microsoft Azure provide powerful services and infrastructure to deploy Docker containers at scale. These cloud providers offer managed services for container orchestration, load balancing, networking, and scaling, making it easier for organizations to handle containerized workloads without worrying about the underlying infrastructure.
In this chapter, we will explore how to deploy Docker containers on three major cloud platforms: AWS, Google Cloud Platform, and Microsoft Azure. We will also discuss how to set up Continuous Integration and Continuous Deployment (CI/CD) pipelines to automate container deployment in the cloud.
11.1 Using Docker with AWS
Amazon Web Services (AWS) is one of the most popular cloud platforms and offers a range of services for deploying and managing Docker containers. AWS provides several tools for container management, including Amazon Elastic Container Service (ECS), Elastic Kubernetes Service (EKS), and AWS Fargate.
11.1.1 Deploying Docker Containers with Amazon ECS
Amazon ECS is a fully managed container orchestration service that simplifies the deployment, management, and scaling of Docker containers. ECS can run Docker containers on a cluster of EC2 instances or on serverless infrastructure using AWS Fargate.
Step 1: Create an ECS Cluster
Before deploying containers, you need to create an ECS cluster. An ECS cluster is a logical grouping of container instances (EC2 instances or Fargate tasks) where your containers will run.
- Log in to the AWS Management Console and navigate to Amazon ECS.
- Select Clusters in the left-hand navigation pane and click Create Cluster.
- Choose a cluster template. For example, select the Networking only option if you plan to use AWS Fargate, or EC2 Linux + Networking if you plan to use EC2 instances.
- Configure the cluster settings:
- Provide a Cluster name.
- Set any optional settings like Instance type and Auto Scaling (for EC2-based clusters).
- Click Create to provision the cluster.
Step 2: Define a Task Definition
In ECS, a task definition is a blueprint for your application. It defines the Docker container image, resource requirements, networking, environment variables, and other configurations for your containers.
- In the ECS console, go to Task Definitions and click Create new Task Definition.
- Choose the Launch Type (either EC2 or Fargate).
- Specify the Task Name and add a container to the task definition.
- Enter the Docker image URL (e.g., from Amazon ECR or Docker Hub).
- Set memory and CPU requirements.
- Define port mappings and environment variables.
- Click Create to save the task definition.
Step 3: Create a Service to Run the Task
A service in ECS ensures that a specified number of task instances are running and manages the deployment of containers.
- In the ECS console, select your Cluster and click Create under the Services tab.
- Choose the Launch Type (EC2 or Fargate).
- Specify the Task Definition and the number of desired tasks (the number of containers to run).
- Configure load balancing if needed (e.g., attaching an Elastic Load Balancer).
- Click Next and then Create Service.
Your Docker containers will now be deployed and running within the ECS cluster.
11.1.2 Using AWS Fargate for Serverless Containers
AWS Fargate is a serverless compute engine for containers that removes the need to manage EC2 instances. With Fargate, you only specify the CPU and memory requirements for your containers, and AWS handles the underlying infrastructure.
- When defining the task definition in ECS, choose Fargate as the launch type.
- Specify your task role and network mode (usually awsvpc for Fargate tasks).
- Select the vPC and subnets where the container will run.
- Deploy your service to Fargate with the same steps as in ECS, but ensure Fargate is selected as the launch type.
With Fargate, AWS automatically provisions, scales, and manages the compute resources required to run your containers.
11.1.3 Scaling Docker Containers in ECS
ECS allows you to scale your containers to handle varying levels of traffic. You can scale your services manually or automatically.
- Manual Scaling: You can manually adjust the number of running tasks in your ECS service through the AWS Management Console or AWS CLI.
- Auto Scaling: ECS can automatically adjust the number of tasks in response to changes in load. You can configure Auto Scaling policies based on metrics like CPU utilization or request count. ECS integrates with CloudWatch to monitor container metrics and trigger scaling actions.
aws ecs update-service --cluster my-cluster --service my-service --desired-count 10
11.1.4 Using Amazon ECR to Store Docker Images
Amazon Elastic Container Registry (ECR) is a fully managed Docker container registry that allows you to store, manage, and deploy Docker images. You can push Docker images to ECR from your local machine or a CI/CD pipeline and use those images in ECS tasks.
Step 1: Create an ECR Repository
- In the AWS Management Console, navigate to Amazon ECR.
- Click Create repository.
- Choose a repository name and click Create repository.
Step 2: Push Docker Images to ECR
Authenticate Docker to the ECR registry:
aws ecr get-login-password --region us-east-1 | docker login --username AWS --password-stdin <aws_account_id>.dkr.ecr.us-east-1.amazonaws.com
Tag your Docker image:
docker tag my-image:latest <aws_account_id>.dkr.ecr.us-east-1.amazonaws.com/my-repository:latest
Push the image to the ECR repository:
docker push <aws_account_id>.dkr.ecr.us-east-1.amazonaws.com/my-repository:latest
Your image is now stored in ECR and can be used in ECS tasks.
11.2 Docker on Google Cloud Platform (GCP)
Google Cloud Platform (GCP) offers several services to deploy and manage Docker containers. The primary service for container orchestration in GCP is Google Kubernetes Engine (GKE), but GCP also provides Cloud Run and Cloud Functions for serverless deployments.
11.2.1 Deploying Docker Containers with Google Kubernetes Engine (GKE)
GKE is a fully managed Kubernetes service that allows you to deploy, manage, and scale containerized applications using Kubernetes.
Step 1: Set Up a GKE Cluster
- Go to the Google Cloud Console and navigate to Kubernetes Engine.
- Click Create Cluster.
- Select the Cluster type (e.g., Standard or Autopilot).
- Configure the cluster settings, such as Cluster name, Location, and Node size.
- Click Create to provision the cluster.
Step 2: Configure kubectl
Once the GKE cluster is created, you need to configure kubectl
to interact with the cluster.
gcloud container clusters get-credentials my-cluster --zone us-central1-a --project my-project-id
Step 3: Deploy Docker Containers on GKE
Create a deployment.yaml file to define your application deployment:
apiVersion: apps/v1 kind: Deployment metadata: name: my-app spec: replicas: 3 selector: matchLabels: app: my-app template: metadata: labels: app: my-app spec: containers: - name: my-container image: gcr.io/my-project/my-image:latest ports: - containerPort: 80
Apply the deployment:
kubectl apply -f deployment.yaml
Expose the deployment using a Service:
kubectl expose deployment my-app --type=LoadBalancer --port=80 --target-port=80
Your Docker container is now running on GKE, and you can access it via the external IP address.
11.2.2 Using Google Cloud Run
Cloud Run is a serverless platform for deploying containerized applications. It abstracts away the underlying infrastructure and automatically scales your application based on incoming traffic.
Build and push your Docker image to Google Container Registry (GCR):
docker tag my-image gcr.io/my-project/my-image:latest docker push gcr.io/my-project/my-image:latest
Deploy the container to Cloud Run:
“`bash gcloud run deploy my-app –image gcr.io/my-project/my-image:latest –platform managed –region us-central1 –allow-
unauthenticated
Cloud Run will automatically handle scaling and expose your application via a URL.
### 11.2.3 Scaling Docker Containers in GKE
In Kubernetes, you can scale your applications by adjusting the **replica count** in the deployment configuration or by using **Horizontal Pod Autoscaler**.
To manually scale your application:
```bash
kubectl scale deployment my-app --replicas=5
To set up an autoscaler:
Create an autoscaler.yaml file:
apiVersion: autoscaling/v2 kind: HorizontalPodAutoscaler metadata: name: my-app-hpa spec: scaleTargetRef: apiVersion: apps/v1 kind: Deployment name: my-app minReplicas: 1 maxReplicas: 10 metrics: - type: Resource resource: name: cpu targetAverageUtilization: 50
Apply the autoscaler:
kubectl apply -f autoscaler.yaml
The Horizontal Pod Autoscaler will automatically adjust the number of pods based on CPU utilization.
11.3 Deploying Containers with Azure
Microsoft Azure provides multiple services for deploying and managing Docker containers, including Azure Kubernetes Service (AKS), Azure Container Instances (ACI), and Azure App Service.
11.3.1 Deploying Docker Containers with Azure Kubernetes Service (AKS)
Azure Kubernetes Service (AKS) is a managed Kubernetes service that simplifies the deployment and management of Kubernetes clusters.
Step 1: Set Up an AKS Cluster
- In the Azure Portal, navigate to Kubernetes Services.
- Click Create and select Azure Kubernetes Service.
- Choose a Resource Group and Cluster name.
- Select a Kubernetes version and Node size.
- Click Review + Create and then Create.
Step 2: Deploy Docker Containers to AKS
Configure
kubectl
to connect to your AKS cluster:az aks get-credentials --resource-group my-rg --name my-aks-cluster
Create a deployment.yaml file for your application:
apiVersion: apps/v1 kind: Deployment metadata: name: my-app spec: replicas: 3 selector: matchLabels: app: my-app template: metadata: labels: app: my-app spec: containers: - name: my-container image: my-container-image:latest ports: - containerPort: 80
Apply the deployment:
kubectl apply -f deployment.yaml
Expose the deployment using a service:
kubectl expose deployment my-app --type=LoadBalancer --port=80 --target-port=80
11.3.2 Using Azure Container Instances (ACI)
Azure Container Instances (ACI) allows you to run Docker containers without the need to manage underlying virtual machines.
Deploy a Docker container to ACI:
az container create --name my-container --image my-image --resource-group my-rg --cpu 1 --memory 1.5
ACI provides a quick and simple way to run containers in Azure with automatic scaling and no need for Kubernetes or VM management.
11.4 CI/CD Pipelines in the Cloud
CI/CD pipelines automate the process of testing, building, and deploying applications. By integrating Docker with CI/CD tools like Jenkins, GitLab CI, Azure DevOps, and GitHub Actions, you can streamline container deployment to the cloud.
11.4.1 Using Jenkins for CI/CD with Docker
Jenkins is an open-source automation server that supports continuous integration and continuous delivery. You can use Jenkins to automate the building and deployment of Docker containers to cloud services like AWS, GCP, and Azure.
Install Jenkins and the Docker plugin.
Configure your Jenkins pipeline to build a Docker image:
pipeline { agent any stages { stage('Build Docker Image') { steps { script { docker.build('my-image') } } } stage('Push to ECR') { steps { script { docker.withRegistry('https://<aws_account_id>.dkr.ecr.us-east-1.amazonaws.com', 'aws-ecr-credentials') { docker.image('my-image').push('latest') } } } } } }
Jenkins will now automatically build, test, and deploy Docker containers.
11.4.2 Using GitHub Actions for Docker CI/CD
GitHub Actions provides an integrated way to automate workflows for building, testing, and deploying Docker containers.
Create a .github/workflows/docker.yml file in your repository:
name: Build and Deploy Docker image on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Check out repository uses: actions/checkout@v2 - name: Set up Docker Buildx uses: docker/setup-buildx-action@v2 - name: Build Docker image run: | docker build -t my-image . - name: Push Docker image to Docker Hub run: | docker login -u ${{ secrets.DOCKER_USERNAME }} -p ${{ secrets.DOCKER_PASSWORD }} docker push my-image
This workflow will automatically build and push Docker images to Docker Hub upon a push to the main
branch.
11.4.3 Using GitLab CI/CD for Docker
GitLab CI/CD is another powerful tool for automating Docker-based workflows.
Create a .gitlab-ci.yml file in your repository:
stages: - build - deploy build: script: - docker build -t my-image . deploy: script: - docker push my-image
GitLab will automatically build and push Docker images as part of the pipeline.
Conclusion
Deploying Docker containers in the cloud is a powerful way to ensure that your applications are scalable, portable, and easy to manage. AWS, GCP, and Azure provide robust services for deploying, scaling, and managing Docker containers, whether you're using ECS, GKE, AKS, or serverless services like AWS Fargate or Google Cloud Run.
Additionally, CI/CD pipelines streamline the process of testing, building, and deploying containers to cloud environments. By leveraging the capabilities of Jenkins, GitHub Actions, and GitLab CI/CD, developers can automate container deployments and ensure a consistent and reliable delivery process.
With the growing adoption of containers and microservices architectures, understanding how to deploy and manage Docker containers in the cloud is becoming an essential skill for developers and DevOps engineers.
Monitoring and Logging with Docker
In today's rapidly evolving world of DevOps and microservices, containers have emerged as a game-changing technology. Docker, in particular, has become the de facto standard for containerization. With Docker containers, developers can easily package applications and their dependencies into a single, portable unit, making it easier to run applications across different environments. However, with the proliferation of Docker containers comes the need for robust monitoring and logging strategies. In this chapter, we'll explore how to effectively monitor and log Docker containers, ensuring the reliability and performance of your containerized applications.
12.1 Docker Monitoring Tools
Monitoring Docker containers is essential to ensure that your applications are running smoothly. Docker containers, by their nature, are ephemeral, meaning they can be created and destroyed on-demand. This transient nature adds a layer of complexity to monitoring, as you need to track the health, performance, and resource utilization of containers in real-time.
There are several Docker monitoring tools available that provide valuable insights into the health, performance, and resource utilization of your containers. Let’s look at some of the most popular ones.
12.1.1 Docker Stats Command
One of the most basic ways to monitor Docker containers is by using the built-in docker stats
command. This command provides real-time metrics about the resource usage of containers, including CPU usage, memory usage, network I/O, and disk I/O. Here’s how you can use it:
docker stats
This will output a table with the following columns:
- CONTAINER ID: The ID of the container.
- NAME: The name of the container.
- CPU %: The percentage of CPU the container is using.
- MEM USAGE / LIMIT: The amount of memory the container is using, along with the total available memory.
- MEM %: The percentage of the container’s memory usage relative to the available memory.
- NET I/O: The amount of data the container has sent and received over the network.
- BLOCK I/O: The amount of data the container has read and written to disk.
- PIDS: The number of processes running inside the container.
Example:
docker stats --no-stream
The --no-stream
flag tells Docker to output a single snapshot of the stats, instead of continuously updating.
While the docker stats
command is useful for quick checks, it’s limited in scope and doesn’t provide long-term data. For more advanced monitoring, you’ll need a dedicated monitoring tool.
12.1.2 Prometheus and Grafana
Prometheus is an open-source monitoring and alerting toolkit, and it is one of the most widely used tools for monitoring Docker containers. It collects and stores metrics as time-series data and is highly suited for environments with dynamic, ephemeral infrastructure such as Docker.
To monitor Docker containers with Prometheus, you can set up a Prometheus server to scrape metrics from a Docker exporter.
Steps to set up Prometheus for Docker:
- Install Prometheus and Docker Exporter: Prometheus itself doesn't collect Docker metrics directly. For this, we need a Prometheus exporter for Docker.
# Run Prometheus and Docker exporter as containers
docker run -d -p 9090:9090 --name=prometheus prom/prometheus
docker run -d -p 8080:8080 --name=docker_exporter -v /var/run/docker.sock:/var/run/docker.sock -e TELEMETRY_PORT=8080 --restart always quay.io/prometheus/dockerd-exporter
- Configure Prometheus to scrape metrics: Update the Prometheus configuration file to scrape the Docker exporter.
# prometheus.yml
scrape_configs:
- job_name: 'docker'
static_configs:
- targets: ['docker_exporter:8080']
- Launch Prometheus: After configuration, you can launch Prometheus using Docker.
docker run -d -p 9090:9090 --name=prometheus -v /path/to/prometheus.yml:/etc/prometheus/prometheus.yml prom/prometheus
- Visualize Metrics with Grafana: Once Prometheus is collecting metrics, you can visualize the data using Grafana, a powerful open-source data visualization tool.
To do this:
- Install Grafana using Docker.
- Connect Grafana to Prometheus as a data source.
- Create dashboards to visualize container performance metrics, such as CPU usage, memory consumption, disk I/O, and network traffic.
docker run -d -p 3000:3000 --name=grafana grafana/grafana
12.1.3 cAdvisor
cAdvisor (short for "Container Advisor") is an open-source tool developed by Google that provides container-level monitoring. cAdvisor collects information about the performance of containers, such as CPU usage, memory usage, file system usage, and network statistics.
You can run cAdvisor as a Docker container, and it will automatically collect and expose metrics for each running Docker container on your host.
docker run -d --name=cadvisor -p 8080:8080 --volume=/:/rootfs:ro --volume=/var/run/docker.sock:/var/run/docker.sock:ro --volume=/sys:/sys:ro --volume=/var/lib/docker/:/var/lib/docker:ro google/cadvisor:latest
Once running, you can navigate to http://localhost:8080
to view cAdvisor’s web interface, which provides a real-time overview of all running containers.
12.1.4 Docker Enterprise Monitoring
For enterprise-level Docker deployments, Docker Enterprise Edition (EE) provides built-in monitoring capabilities. Docker EE includes an integrated monitoring and alerting system for containers. This can be managed through Docker’s own graphical interface (Docker Enterprise Control Plane) or through integrations with third-party monitoring tools.
Docker EE also integrates with various cloud platforms, including AWS, Azure, and Google Cloud, offering a centralized monitoring and alerting platform for containerized applications deployed on these platforms.
12.2 Log Aggregation and Analysis
Logging is another essential component of monitoring Docker containers. Since containers are designed to be ephemeral, centralized log aggregation and analysis are critical to ensure you don’t lose important logs when containers stop or are restarted.
There are several strategies and tools available to help you aggregate and analyze logs from your Docker containers.
12.2.1 Docker Logs Command
Each Docker container automatically generates logs, which can be viewed using the docker logs
command. The docker logs
command displays the standard output (stdout) and standard error (stderr) from the container, including anything written to the console during the container's execution.
To view the logs of a running container:
docker logs <container_id or container_name>
For real-time logging, you can use the -f
flag to "follow" the logs:
docker logs -f <container_id or container_name>
You can also combine this with filtering options such as --since
or --tail
to limit the log output:
docker logs --since="2024-11-01T00:00:00" --tail 100 <container_id>
However, while useful for debugging individual containers, relying solely on docker logs
is not practical for larger environments where many containers may be running. For this, we need a log aggregation tool.
12.2.2 ELK Stack (Elasticsearch, Logstash, Kibana)
The ELK stack is a powerful set of tools for centralized logging, aggregation, and analysis. It consists of the following components:
- Elasticsearch: A search and analytics engine that stores and indexes logs.
- Logstash: A log pipeline tool that collects, processes, and forwards logs.
- Kibana: A data visualization and exploration tool used to view and analyze logs.
To set up centralized logging with the ELK stack, you need to configure your Docker containers to send logs to Logstash, which will process and forward them to Elasticsearch for storage and indexing. Kibana can then be used to visualize the logs.
Here’s a basic setup:
- Run Elasticsearch and Kibana:
docker run -d --name=elasticsearch -p 9200:9200 docker.elastic.co/elasticsearch/elasticsearch:7.10.0
docker run -d --name=kibana -p 5601:5601 docker.elastic.co/kibana/kibana:7.10.0
- Run Logstash:
You can configure Logstash to receive logs from Docker containers. First, create a logstash.conf
configuration file:
input {
tcp {
port => 5000
codec => json
}
}
output {
elasticsearch {
hosts => ["http://elasticsearch:9200"]
index => "docker-logs-%{+YYYY.MM.dd}"
}
}
Then, run Logstash with the configuration:
docker run -d --name=logstash -v /path/to/logstash.conf:/usr/share/logstash/pipeline/logstash.conf docker.elastic.co/logstash/logstash:7.10.0
- Configure Docker to send logs to Logstash:
Edit your Docker daemon settings to use the gelf
log driver, which sends logs in a format that Logstash understands. Add the following to your daemon.json
:
{
"log-driver": "gelf",
"log-opts": {
"gelf-address": "tcp://logstash:5000"
}
}
Now your Docker containers will send logs to Logstash, which
will forward them to Elasticsearch for indexing.
- View logs in Kibana: Once Elasticsearch has indexed the logs, you can use Kibana to create visualizations, dashboards, and alerts based on your log data.
12.2.3 Fluentd
Fluentd is another popular log aggregation tool that can collect logs from various sources, including Docker containers. Fluentd is often used as an intermediary between Docker and other logging backends such as Elasticsearch or a cloud-based log storage system.
To use Fluentd with Docker:
- Run Fluentd:
docker run -d -p 24224:24224 --name=fluentd fluent/fluentd:v1.12-1
- Configure Docker to use Fluentd:
Edit the daemon.json
file to set the fluentd
log driver:
{
"log-driver": "fluentd",
"log-opts": {
"fluentd-address": "fluentd:24224"
}
}
Fluentd will forward logs to its output plugin, such as Elasticsearch, Google Cloud Storage, or other backends.
12.2.4 Cloud-Based Log Aggregation
In modern cloud-native environments, many organizations rely on cloud-based log aggregation services. AWS, Google Cloud, and Azure all offer integrated log aggregation solutions:
- AWS CloudWatch Logs: Collects, monitors, and stores log files from your containers.
- Google Cloud Logging: Provides a unified platform for managing logs across services.
- Azure Monitor Logs: Offers similar functionality for Azure-hosted containers.
These services often come with additional features such as managed alerting, log retention policies, and easy integration with other cloud services.
12.3 Health Checks for Docker Containers
Health checks are an important aspect of container management. They allow Docker to automatically detect when a container is unhealthy and take action accordingly, such as restarting the container or notifying administrators.
Docker supports defining health checks for containers using the HEALTHCHECK
instruction in a Dockerfile or using the --health-cmd
option when running a container.
12.3.1 Defining a Health Check in a Dockerfile
You can define a health check in your Dockerfile by using the HEALTHCHECK
instruction. For example:
FROM node:14
WORKDIR /app
COPY . .
RUN npm install
HEALTHCHECK CMD curl --fail http://localhost:3000/health || exit 1
In this example, the health check sends a curl
request to http://localhost:3000/health
. If the server responds successfully, the container is considered healthy. If the request fails (e.g., the server is down), Docker marks the container as unhealthy.
12.3.2 Health Check Parameters
The HEALTHCHECK
instruction has several optional parameters:
- CMD: The command to run to check the health of the container. If the command exits with a non-zero status, the container is considered unhealthy.
- INTERVAL: The time between running health checks (default is 30 seconds).
- TIMEOUT: The time to wait before considering a health check as failed (default is 30 seconds).
- START_PERIOD: The time Docker will wait after the container starts before performing the first health check.
- RETRIES: The number of consecutive failures before Docker considers the container unhealthy (default is 3).
Example with parameters:
HEALTHCHECK --interval=10s --timeout=5s --retries=3 CMD curl --fail http://localhost:3000/health || exit 1
12.3.3 Checking Health Status
You can check the health status of a container using the docker inspect
command:
docker inspect --format '{{.State.Health.Status}}' <container_name_or_id>
This will return the health status (healthy
, unhealthy
, or starting
).
12.4 Scaling Docker Containers
Scaling Docker containers is an important part of ensuring that your applications are able to handle increased load. Docker itself doesn’t natively provide automatic scaling, but when used with orchestration tools like Docker Compose, Docker Swarm, or Kubernetes, you can easily scale your containers up or down to meet demand.
12.4.1 Scaling with Docker Compose
Docker Compose allows you to define and run multi-container Docker applications. It also supports scaling services based on your needs. Here's how to scale a service in Docker Compose.
- Define a Service in
docker-compose.yml
:
version: '3'
services:
web:
image: nginx
ports:
- "8080:80"
- Scale the Service:
You can scale the service using the --scale
flag when running docker-compose up
:
docker-compose up --scale web=3
This command will create three replicas of the web
service, effectively scaling the application.
12.4.2 Scaling with Docker Swarm
Docker Swarm is Docker's native clustering and orchestration tool, which allows you to scale containers across multiple nodes.
- Initialize Docker Swarm:
docker swarm init
- Deploy a Service:
docker service create --name web --replicas 3 -p 8080:80 nginx
This command creates a service named web
with 3 replicas.
- Scale the Service:
You can scale the service at any time by updating the number of replicas:
docker service scale web=5
This will scale the web
service to 5 replicas.
12.4.3 Auto-Scaling with Kubernetes
Kubernetes provides advanced capabilities for automatically scaling applications based on resource usage or custom metrics. Kubernetes uses Horizontal Pod Autoscaler to automatically scale the number of pods in a deployment.
To enable auto-scaling, you need to set up a deployment and define an autoscaler.
- Create a Deployment:
kubectl create deployment nginx --image=nginx
- Create an Autoscaler:
kubectl autoscale deployment nginx --cpu-percent=50 --min=1 --max=10
This command sets up an autoscaler that will scale the nginx
deployment between 1 and 10 replicas based on CPU usage.
In conclusion, Docker monitoring, logging, and scaling are essential aspects of containerized application management. Whether you're tracking resource usage with tools like docker stats
, aggregating logs with the ELK stack, or implementing health checks, these practices help ensure that your applications run smoothly in production. Furthermore, scaling your Docker containers with tools like Docker Compose, Docker Swarm, or Kubernetes enables your applications to meet demand and handle traffic fluctuations effectively.
Sure! Here's an expanded version of the chapter on Docker Security Best Practices, complete with detailed explanations and code examples.
Docker Security Best Practices
Docker has revolutionized how we build, ship, and run applications, making it easier to package applications along with all their dependencies in containers. However, with the immense flexibility and speed that Docker offers, it also brings a new set of security challenges. Securing Docker containers is critical for ensuring that your applications run safely in a production environment.
This chapter will explore Docker security best practices across four important areas:
- Securing Docker Images
- Docker User Management and Permissions
- Running Containers as Non-root Users
- Network Security in Docker
13.1 Securing Docker Images
What is a Docker Image?
A Docker image is a blueprint for creating Docker containers. It contains everything needed to run an application — including the code, runtime, libraries, and environment variables. Since Docker images can be publicly shared and pulled from repositories like Docker Hub or private registries, they can sometimes pose security risks if not properly vetted.
Best Practices for Securing Docker Images
Use Official Images or Trusted Sources
One of the first steps in securing Docker images is ensuring that you are using trusted sources. Docker Hub provides a large repository of pre-built images, but not all of them are secure. Always prefer official or well-maintained images, and check the Docker Hub page for metadata like usage statistics, the latest update date, and reviews.docker pull ubuntu:20.04 # Official Ubuntu image
Minimize the Attack Surface
Use minimal base images. For example, instead of using a full-fledged operating system image likeubuntu
ordebian
, you can use lightweight images likealpine
orbusybox
. These images contain only the bare essentials, which reduces the number of potential vulnerabilities.# Using a minimal Alpine image FROM alpine:3.15
Scan Images for Vulnerabilities
Regularly scan Docker images for vulnerabilities. Docker provides a security scanning tool that can identify potential vulnerabilities in your images. Docker Desktop also comes with integrated scanning features to warn you about known vulnerabilities.Additionally, tools like Clair (by CoreOS) or Trivy can be used to scan container images for vulnerabilities.
Example with Trivy:
trivy image your_image:latest
Use Multi-stage Builds
When creating custom images, use multi-stage builds to reduce the size of the final image. By separating the build environment from the runtime environment, you can ensure that unnecessary tools (such as compilers) are excluded from the production image, which reduces the attack surface.Example of a multi-stage build:
# Build stage FROM node:16 as build-stage WORKDIR /app COPY . . RUN npm install RUN npm run build # Final stage FROM node:16-slim WORKDIR /app COPY --from=build-stage /app/dist /app CMD ["node", "dist/app.js"]
Sign and Verify Images
Docker Content Trust (DCT) allows you to sign images and verify the integrity and authenticity of images before pulling them. Enabling Docker Content Trust ensures that only signed images are pulled, preventing the use of unauthorized or tampered images.Enable Docker Content Trust:
export DOCKER_CONTENT_TRUST=1
Use .dockerignore to Exclude Sensitive Files
When building Docker images, it's important to ensure that sensitive files, such as.env
files, keys, or credentials, are not included in the final image. This can be done by specifying these files in a.dockerignore
file.Example
.dockerignore
:.env .git *.pem
Update Images Regularly
Just like any software, Docker images should be updated to include the latest security patches. Regularly pull the latest version of images from the source or rebuild custom images to ensure you are using the most up-to-date, secure versions.docker pull your_image:latest
Avoid Running Containers with Unnecessary Privileges
Avoid running containers with unnecessary privileges like root, and restrict capabilities as much as possible. You can remove unused capabilities or set specific ones using Docker’s--cap-drop
and--cap-add
flags.docker run --cap-drop=ALL --cap-add=NET_BIND_SERVICE nginx
13.2 Docker User Management and Permissions
User Management in Docker
In Docker, the user who runs the Docker daemon has full administrative control over all containers and images. If the Docker daemon is compromised, the attacker gains full control of the system. Therefore, proper user management and permissions are crucial to Docker security.
Best Practices for Docker User Management
Limit Docker Daemon Access
The Docker daemon runs with root privileges, which means any user with access to the Docker socket (/var/run/docker.sock
) can execute Docker commands and have root access to the host machine. To limit this, you should avoid giving unnecessary users access to Docker commands.- Add users to the
docker
group (if necessary) but ensure only trusted users have access.
sudo usermod -aG docker your_username
- Add users to the
Use Least Privilege Principle
Always follow the least privilege principle. Only grant users the minimum permissions they need to perform their tasks. For example, avoid running Docker containers as root unless absolutely necessary.Set User Permissions for Dockerfiles
In Dockerfiles, you can specify the user that the container will run as by using theUSER
directive. This reduces the need for running containers as root, minimizing potential attack vectors.Example:
FROM ubuntu RUN groupadd -r appuser && useradd -r -g appuser appuser USER appuser
Separate Docker Users and System Users
Avoid using system users (such asroot
orwww-data
) within Docker containers unless required. Instead, create dedicated users for your applications inside the Docker container.Example of adding a non-root user to a Dockerfile:
RUN groupadd -g 1001 appuser && useradd -r -u 1001 -g appuser appuser
Restrict Access to Docker Socket
The Docker socket (/var/run/docker.sock
) allows access to the Docker daemon and the host system. Make sure that only trusted users and services can access this socket. One way to restrict access is to use Unix socket permissions or set up an access control policy.chmod 660 /var/run/docker.sock
Audit and Monitor User Activity
Regularly audit user activity on the Docker daemon. This can be done by enabling logging and using external monitoring tools like Auditd, Sysdig, or Docker’s own logging capabilities to track who is performing actions.
13.3 Running Containers as Non-root Users
Why Run Containers as Non-root Users?
Running containers as root can give attackers complete access to the host machine if the container is compromised. Therefore, always strive to run containers with a non-root user, particularly in production environments.
Best Practices for Running Containers as Non-root Users
Create and Use Non-root Users
By default, containers may run as root, but you can override this behavior by specifying a non-root user in your Dockerfile.Example:
# Create a non-root user RUN groupadd -g 1001 appuser && useradd -r -u 1001 -g appuser appuser USER appuser
Use the
USER
Directive
TheUSER
directive in a Dockerfile specifies the user to run the application. Running as a non-root user inside the container reduces the attack surface and ensures that the container cannot compromise the host system if compromised.Example:
FROM node:14 RUN useradd -m myappuser USER myappuser
Set File Permissions Appropriately
When creating or modifying files inside a container, ensure that the files are owned by the non-root user. This prevents root from modifying sensitive files or exploiting file permissions in a compromised container.Example:
RUN chown -R appuser:appuser /app
Run Containers with the
--user
Flag
If you need to run a container as a specific user, use the--user
flag withdocker run
to specify the user ID (UID) and group ID (GID).Example:
docker run --user 1001:1001 my_image
Use Security Profiles (Seccomp, AppArmor, SELinux)
Docker supports several security profiles like Seccomp, AppArmor, and SELinux to enforce security policies. These profiles restrict the system calls and operations that a container can perform. Enabling these profiles can significantly limit the damage a compromised container can cause.Example:
docker run --security-opt seccomp=default.json my_image
Use Docker Content Trust (DCT)
Enabling Docker Content Trust ensures that only signed images are pulled and run, preventing the use of potentially compromised or tampered images.export DOCKER_CONTENT_TRUST=1
13.4 Network Security in Docker
Docker Networking Overview
Docker containers are isolated from each other and from the host system by default, but containers can communicate over a virtual network. Docker provides various networking modes, such as bridge
, host
, none
, and overlay
, to control how containers communicate with each other and the outside world.
Best Practices for Network Security in Docker
Use Isolated Networks
Create isolated networks for containers to limit communication to only necessary containers. By default, containers are attached to thebridge
network, but you can create custom networks for greater control.Example:
docker network create --driver bridge isolated_network
Use the
--icc
Flag to Control Inter-container Communication
By default, containers on the same network can communicate with each other. If you want to prevent this, use the--icc
(inter-container communication) flag to disable communication between containers.Example:
docker network create --icc=false no-icc-network
Limit Published Ports
Expose only the ports that are absolutely necessary for communication with the outside world. This reduces the attack surface by limiting access to your containers.Example:
docker run -p 8080:80 my_image
Use Firewall Rules for Docker Networks
Docker allows you to define firewall rules that control access between containers and external networks. Use these rules to secure your network and prevent unauthorized access.Example with Docker's default
bridge
network:iptables -A DOCKER-USER -s 192.168.1.0/24 -j DROP
Use Overlay Networks for Multi-host Communication
In a multi-host Docker setup, use overlay networks to securely connect containers across different hosts. Overlay networks encrypt traffic between containers, ensuring secure communication.Example:
docker network create --driver overlay my_overlay_network
Limit Container Communication with
--link
and--add-host
When running containers that need to communicate with each other, avoid using the--link
flag, as it is deprecated. Instead, use DNS names and explicitly define hostnames using the--add-host
option.
This concludes our detailed look at Docker security best practices. Following these guidelines can significantly reduce the risk of vulnerabilities and attacks in Docker environments, ensuring that your containers run securely and efficiently.
Troubleshooting Docker
Docker has revolutionized the way developers create, ship, and run applications, making it easier to deploy applications in a consistent environment. However, like any complex system, Docker can encounter issues, and understanding how to troubleshoot them effectively is crucial for maintaining the reliability and stability of your containers and services. This chapter will provide an in-depth guide to common Docker issues, diagnostic tools and commands, and how to debug container failures.
14.1 Common Docker Issues
When working with Docker, you may encounter a range of problems. These issues can arise at various stages of the container lifecycle, such as building images, running containers, or networking between containers. Below are some of the most common Docker issues that developers and system administrators face.
1. Docker Daemon Not Running
A very basic yet common issue is when the Docker daemon is not running. The Docker daemon is the background service responsible for managing containers on a system. If the daemon isn't running, all Docker commands (like docker ps
, docker run
, or docker build
) will fail.
Symptoms:
- Running any Docker command results in an error saying "Cannot connect to the Docker daemon" or "docker: Cannot connect to the Docker daemon at unix:///var/run/docker.sock."
- Docker services may fail to start when booting the system.
Solutions:
Start Docker Daemon: Use the following command to start the Docker daemon if it is not running.
sudo systemctl start docker
Check Status: To verify if the Docker daemon is running, you can check the service status.
sudo systemctl status docker
Enable Docker at Boot: If Docker is not set to start on boot, you can enable it with:
sudo systemctl enable docker
2. Container Exits Immediately
It is common to see a container start and then exit immediately. This can happen if the command or entrypoint in the container finishes executing or fails unexpectedly.
Symptoms:
- The container starts but stops right away.
- The
docker ps
command does not show the container, butdocker ps -a
shows it with a status of "Exited."
Solutions:
Check Container Logs: You can inspect the logs of the container to understand why it is exiting.
docker logs <container_id>
Interactive Mode for Debugging: If the issue isn't clear from the logs, try running the container interactively to troubleshoot.
docker run -it <image> /bin/bash
Set a Long-Running Command: If you are using a custom image, ensure the entrypoint or command specified in the Dockerfile runs as a long-lived process, such as a web server or database.
3. Container Cannot Connect to External Network
Network connectivity issues between a Docker container and the external world are also common, particularly when the container cannot reach external services like a database or a remote API.
Symptoms:
- Network timeouts or unreachable errors when attempting to connect to services outside of Docker.
- Error messages related to DNS resolution failures, such as
Could not resolve host
.
Solutions:
Check DNS Settings: Ensure that the container's DNS settings are correctly configured. By default, Docker containers use the host's DNS settings.
docker run --dns 8.8.8.8 <image>
Test Network Connectivity: Try running basic network tools (like
ping
orcurl
) inside the container.docker run -it <image> ping google.com
Inspect Docker Network Configuration: Docker uses several network drivers (bridge, host, overlay, etc.). If your container is part of a custom bridge network, ensure that the networking settings are configured correctly. You can check the network configuration with:
docker network inspect <network_name>
4. Volume Mounting Issues
Volume mounting is essential for persisting data across container restarts, but issues can arise when volumes are not properly mounted, causing data to be lost or the application to malfunction.
Symptoms:
- Data does not persist after restarting a container.
- Permission errors when accessing files inside mounted volumes.
Solutions:
Check Volume Mount Path: Verify that the source path on the host is correct and the destination path inside the container is properly specified.
docker run -v /host/path:/container/path <image>
Check Permissions: Ensure the container has the appropriate read/write permissions for the mounted volume.
sudo chmod -R 777 /host/path
Use Named Volumes for Persistence: Named volumes (instead of bind mounts) can often help with portability and prevent some of the permission issues:
docker volume create my_volume docker run -v my_volume:/container/path <image>
5. Out of Memory Errors
Docker containers can run out of memory if not properly configured, especially when dealing with resource-intensive applications.
Symptoms:
- The container is killed due to the application consuming too much memory, often accompanied by a "OOM" (Out Of Memory) message.
- Slow container performance or crashing.
Solutions:
Allocate Memory Limits: You can limit the memory usage of containers by specifying resource limits when running a container:
docker run --memory="500m" <image>
Monitor Resource Usage: Use Docker’s
stats
command to monitor the memory usage of running containers:docker stats
Optimize the Application: Review the application inside the container to identify potential memory leaks or inefficient memory usage.
14.2 Diagnostic Tools and Commands
To effectively troubleshoot Docker, it’s important to know which tools and commands are available to help you diagnose problems. Docker provides a range of diagnostic tools and commands that can give you insight into container status, logs, configuration, and more.
1. Docker Logs
The docker logs
command is one of the most useful tools when diagnosing container issues. It allows you to view the output of a container’s process.
Usage:
docker logs <container_id>
You can also follow the logs in real-time using the -f
flag:
docker logs -f <container_id>
2. Docker Events
The docker events
command provides a real-time stream of events happening in the Docker daemon. This includes container starts, stops, restarts, and other changes to Docker objects.
Usage:
docker events
This command is particularly useful when you want to monitor the health of containers and track events that could lead to failures.
3. Docker Inspect
The docker inspect
command provides detailed information about containers, images, volumes, and networks. It outputs a JSON object that includes metadata about the object.
Usage:
To inspect a container:
docker inspect <container_id>
For a network:
docker network inspect <network_name>
For a volume:
docker volume inspect <volume_name>
This command is extremely useful for gathering low-level details about how Docker is configured and helps in understanding configuration mismatches or resource limitations.
4. Docker Stats
The docker stats
command shows real-time statistics about resource usage (CPU, memory, network, and I/O) of containers.
Usage:
docker stats
To monitor a specific container:
docker stats <container_id>
This tool is crucial for identifying resource bottlenecks and containers that are consuming excessive CPU or memory.
5. Docker Top
The docker top
command shows the processes running inside a container, similar to the ps
command in Linux.
Usage:
docker top <container_id>
This command is useful for identifying runaway processes or resource hogs inside a container.
6. Docker Diff
The docker diff
command shows the changes made to the filesystem of a container. It reports added, modified, or deleted files, which is helpful for debugging issues related to file corruption or unexpected changes.
Usage:
docker diff <container_id>
14.3 Debugging Container Failures
When a container fails, it is important to diagnose the cause systematically. Below are several strategies for debugging container failures.
1. Start with Logs
The first step in debugging any container failure is to check the logs. As mentioned earlier, the docker logs
command can give you insight into what went wrong. Pay close attention to any error messages or stack traces that may point to a specific issue in the application or environment.
2. Reproduce the Issue Locally
If the container is failing in a production environment, try to reproduce the issue locally using the same image and configuration. Running the container in an interactive mode (docker run -it
) can help you see what happens during container startup and pinpoint the failure.
3. Check Resource Usage
If a container fails due to resource exhaustion (such as running out of memory or CPU), use tools like docker stats
and docker top
to monitor resource usage. These tools can help you determine whether the container is consuming too many resources and whether resource limits need to be adjusted.
4. Check Dependencies and Networking
Some containers may fail due to issues with external dependencies or networking. Ensure that the container can access the necessary services, such as databases or external APIs. Use tools like docker network inspect
to confirm that networking is configured correctly.
5. Use a Different Base Image
Sometimes the issue could be related to the base image itself. Try building the container from a different base image, or make sure that the base image you are using is up-to-date and free of known issues. You can also try using a different version of the same image.
6. Run Containers with Debugging Tools
For more complex debugging, you can run containers with debugging tools installed (such as strace
, gdb
, or lsof
). This will allow you to trace system calls, debug processes, or inspect open files and network connections.
In conclusion, troubleshooting Docker can seem challenging, but with the right tools and strategies, you can quickly identify and resolve common issues. Understanding how to access logs, inspect configuration, monitor resource usage, and diagnose container failures is crucial for maintaining stable and reliable containerized applications.
Advanced Docker Techniques
Docker has revolutionized the way developers deploy and manage applications. It offers the ability to encapsulate applications and their dependencies in lightweight, portable containers. This chapter dives deeper into some advanced Docker techniques, including multi-stage builds, Docker's use in microservices, building custom networks, and using Docker in machine learning workflows. Each of these techniques enhances the power and flexibility of Docker, providing you with tools to build more efficient and scalable applications.
15.1 Multi-Stage Builds
What are Multi-Stage Builds?
Multi-stage builds in Docker allow you to create smaller, more efficient images by separating the build environment from the runtime environment. In a traditional Dockerfile, you would install all dependencies, build your application, and end up with a final image that contains all the necessary binaries and libraries, even those needed only for building the application. This could result in a bloated image.
With multi-stage builds, you can define multiple stages in a single Dockerfile. Each stage has its own FROM
statement, allowing you to pull different base images for each stage. This lets you install build dependencies in an earlier stage, build the application, and then copy only the necessary artifacts into the final image, leaving behind unnecessary build dependencies.
Benefits of Multi-Stage Builds
- Smaller Final Images: By eliminating build dependencies from the final image, multi-stage builds can drastically reduce the size of the image.
- Cleaner Dockerfiles: Multi-stage builds allow you to separate concerns more clearly in your Dockerfile, making it easier to understand and maintain.
- Faster Build Times: By optimizing the Dockerfile and its build process, you can reduce the number of layers and improve caching, leading to faster builds.
Example: Using Multi-Stage Builds
Let's look at an example where we build a Node.js application using multi-stage builds.
# Stage 1: Build stage
FROM node:16 AS builder
# Set the working directory inside the container
WORKDIR /app
# Install dependencies
COPY package.json package-lock.json ./
RUN npm install
# Copy the application source code
COPY . .
# Build the application (for example, for production)
RUN npm run build
# Stage 2: Final stage (runtime)
FROM node:16-slim
# Set the working directory inside the container
WORKDIR /app
# Copy only the necessary files from the builder stage
COPY --from=builder /app/build /app/build
COPY --from=builder /app/node_modules /app/node_modules
COPY --from=builder /app/package.json /app/package.json
# Expose the port the app will run on
EXPOSE 3000
# Start the application
CMD ["npm", "start"]
Explanation:
Stage 1: Builder Stage
- We start by using the official Node.js image
node:16
and give it an aliasbuilder
. - We set up the working directory inside the container and copy the
package.json
andpackage-lock.json
files, followed by runningnpm install
to install dependencies. - The application source code is then copied, and
npm run build
is executed to create a production build of the app.
- We start by using the official Node.js image
Stage 2: Runtime Stage
- For the final runtime image, we switch to a smaller Node.js image (
node:16-slim
) to reduce the final image size. - We use the
COPY --from=builder
command to copy only the necessary build artifacts (likenode_modules
,package.json
, and the build directory) from the builder stage. - Finally, we expose the appropriate port and set the default command to start the application.
- For the final runtime image, we switch to a smaller Node.js image (
This Dockerfile will produce a smaller image since it doesn’t include unnecessary build dependencies like development tools or source code files in the final image.
Best Practices for Multi-Stage Builds
- Use Lightweight Base Images: For runtime stages, use minimal images (like
node:slim
,alpine
, or other optimized images). - Minimize the Number of Layers: Each command in a Dockerfile creates a new layer. Combine related commands using
&&
to reduce the number of layers. - Leverage Cache: Docker caches each layer, so structure the Dockerfile in a way that the layers that change less frequently (like installing dependencies) come earlier in the file to take advantage of caching.
15.2 Docker and Microservices
What are Microservices?
Microservices is an architectural style that breaks down an application into small, loosely coupled, independently deployable services. Each service is focused on a single business capability and can be developed, deployed, and scaled independently of other services.
With microservices, you often deal with multiple containers that need to communicate with each other, share data, and sometimes scale independently. Docker is an excellent fit for this architecture since it provides isolated environments for each microservice, making it easier to deploy and manage these services.
Why Docker and Microservices?
- Isolation: Each microservice runs in its own container, ensuring that it is isolated from others. This prevents dependencies from clashing.
- Scalability: Docker makes it easy to scale services horizontally by adding or removing containers based on demand.
- Portability: Docker ensures that microservices run the same way in development, testing, and production environments.
- Continuous Integration/Continuous Deployment (CI/CD): Docker supports automated workflows for building, testing, and deploying microservices.
Example: Running Multiple Microservices with Docker Compose
In a microservices architecture, you might have several services that interact with each other. Docker Compose is a tool for defining and running multi-container Docker applications. Here's an example of how you might set up a microservices system using Docker Compose.
Let's assume we have two microservices:
- Web: A Node.js application that serves an API.
- Database: A MySQL database.
We'll define a docker-compose.yml
file to manage these services.
version: '3.8'
services:
web:
image: node:16
container_name: web
build:
context: ./web
ports:
- "3000:3000"
depends_on:
- db
networks:
- app-network
db:
image: mysql:5.7
container_name: db
environment:
MYSQL_ROOT_PASSWORD: example
ports:
- "3306:3306"
networks:
- app-network
networks:
app-network:
driver: bridge
Explanation:
Web Service:
- We define the
web
service using thenode:16
image, which will run a Node.js app. - The service depends on the
db
service, meaning Docker Compose will start thedb
service first. - The
build
context is set to./web
, which means Docker will look for aDockerfile
inside theweb
directory to build the image. - The service exposes port 3000 on the host machine, so you can access the API at
localhost:3000
.
- We define the
DB Service:
- We define the
db
service using themysql:5.7
image. - We set an environment variable for the MySQL root password.
- The MySQL database will be available on port 3306 on the host machine.
- We define the
Networking:
- We define a custom network
app-network
using thebridge
driver. Both theweb
anddb
services are connected to this network, allowing them to communicate with each other.
- We define a custom network
With this setup, you can run the entire application with a single command:
docker-compose up
This will start both the web and database containers, and the web service can communicate with the MySQL database using the hostname db
.
Scaling Microservices
Docker Compose also allows you to scale services easily. For example, if you want to scale the web
service to handle more traffic, you can use the --scale
flag:
docker-compose up --scale web=3
This will start three instances of the web
service, allowing you to handle more requests.
15.3 Building Custom Docker Networks
What are Docker Networks?
Docker containers can communicate with each other through Docker networks. By default, all containers are attached to a default network called bridge
. However, creating custom networks allows you to fine-tune communication between containers and enhance security and isolation.
There are different types of networks in Docker:
- Bridge: The default network driver. Suitable for standalone containers that need to communicate.
- Host: The container shares the host’s network stack. This is often used for performance-critical applications.
- Overlay: Used for multi-host communication. Ideal for Docker Swarm or Kubernetes clusters.
- None: No networking. Containers with this network cannot communicate with others.
Why Build Custom Networks?
- Isolation: Custom networks allow you to isolate containers, ensuring that only those on the same network can communicate.
- Security: With custom networks, you can control the communication paths between services, enhancing security.
- Name Resolution: Docker provides DNS resolution between containers on the same custom network, which makes service discovery easier.
Example: Creating Custom Networks
Let's say we want to create two custom networks for different purposes. We’ll create a frontend
network for web containers and a backend
network for database containers.
# Create custom networks
docker network create frontend
docker network create backend
Now, let’s modify the docker-compose.yml
file to use these networks.
version: '3.8'
services:
web:
image: node:16
container_name: web
build:
context: ./web
ports:
- "3000:3000"
networks:
- frontend
db:
image: mysql:5.7
container_name: db
environment:
MYSQL_ROOT_PASSWORD: example
networks:
- backend
networks:
frontend:
driver: bridge
backend:
driver: bridge
Explanation:
Web Service:
- The
web
service is connected to thefrontend
network, meaning it can communicate with other frontend services (like an API server).
- The
DB Service:
- The
db
service is connected to thebackend
network, isolating it from thefrontend
network.
- The
This configuration allows you to segment your network traffic and ensure that only services that need to communicate with each other can do so.
15.4 Using Docker with Machine Learning
Why Use Docker for Machine Learning?
Machine learning (ML) workflows involve a variety of tools, libraries, and frameworks that need to be consistently configured across different environments. Docker provides an easy way to containerize these dependencies and create reproducible environments for ML projects.
Benefits of Docker for ML
- Reproducibility: Docker ensures that your model and environment can be reproduced across machines, making it easier to share and collaborate.
- Environment Isolation: Each ML project can run in its own container, ensuring that dependencies for one project don’t conflict with others.
- Scalability: With Docker, you can scale ML models by deploying them as services in a microservices architecture, or use Docker Swarm/Kubernetes for large-scale distributed workloads.
Example: Containerizing a Machine Learning Model
Let’s containerize a simple machine learning model built with Python and scikit-learn.
Step 1: Create the ML Script
We'll create a script train_model.py
that trains a simple logistic regression model.
import pickle
from sklearn.datasets import load_iris
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load dataset
iris = load_iris()
X = iris.data
y = iris.target
# Split the dataset into training and testing
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train the model
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Print accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Model Accuracy: {accuracy}")
# Save the model
with open("model.pkl", "wb") as f:
pickle.dump(model, f)
Step 2: Create the Dockerfile
Now, let's create a Dockerfile
to containerize this script.
# Use a Python base image
FROM python:3.8-slim
# Set the working directory
WORKDIR /app
# Install necessary dependencies
COPY requirements.txt .
RUN pip install -r requirements.txt
# Copy the Python script into the container
COPY train_model.py .
# Set the entrypoint for the container
CMD ["python", "train_model.py"]
Step 3: Create the Requirements File
The requirements.txt
file lists the dependencies needed for our ML model.
scikit-learn==0.24.2
numpy==1.21.0
Step 4: Build and Run the Docker Container
To build the Docker image, run:
docker build -t ml-model .
Once the image is built, run the container:
docker run --rm ml-model
This will execute the train_model.py
script inside the container, train the model, and print the accuracy.
Step 5: Share and Deploy the Model
Once the model is trained and saved in the container, you can deploy it in a production environment, or share it with other teams. For example, you can create an API endpoint using Flask to serve the model and deploy it with Docker.
from flask import Flask, request, jsonify
import pickle
app = Flask(__name__)
# Load the trained model
with open("model.pkl", "rb") as f:
model = pickle.load(f)
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json()
prediction = model.predict([data['features']])
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0')
In this example, you can send POST requests with feature data to the /predict
endpoint, and the model will return predictions.
These advanced Docker techniques will help you streamline your workflows, improve scalability, and maintain cleaner, more efficient Dockerfiles for microservices, machine learning, and other complex applications.
The Future of Docker and Containers
Docker revolutionized how we approach software development, deployment, and management, providing a lightweight, portable, and consistent way of running applications across diverse environments. However, as technology continues to evolve, Docker and containerization are adapting to new challenges and use cases. In this chapter, we'll explore the future of Docker and containers by examining key emerging trends like Kubernetes, edge computing, serverless computing, and the ongoing evolution of containerization itself.
16.1 Docker in the Age of Kubernetes
The Rise of Kubernetes
Kubernetes, or K8s, has quickly become the de facto orchestrator for containerized applications. Originally created by Google, it provides a platform to automate the deployment, scaling, and management of containerized applications across a cluster of machines. While Docker serves as the container runtime, Kubernetes adds a layer of complexity by managing containers at scale.
Historically, Docker containers could be run on individual servers or in small groups, but scaling containers manually becomes increasingly difficult as applications grow. Kubernetes solves this problem by abstracting away the underlying infrastructure, enabling developers to focus on their applications rather than managing individual containers.
Docker and Kubernetes: Complementary Technologies
Although Kubernetes has gained significant popularity, it doesn’t replace Docker. Instead, Docker and Kubernetes work hand-in-hand, with Docker providing the container runtime and Kubernetes handling the orchestration of those containers. Docker’s role is to ensure that the application and its dependencies are bundled into a container image, while Kubernetes handles scaling, networking, storage, and fault tolerance.
In Kubernetes, Docker containers are deployed in units called "pods," which can contain one or more containers that are tightly coupled. Kubernetes takes care of scheduling pods on nodes in the cluster, managing resource allocation, and ensuring that the right number of replicas of a pod are running. This makes Kubernetes a powerful tool for managing large-scale containerized applications, but it’s Docker that provides the containerization at the core.
Docker in Kubernetes Workflows
In a Kubernetes setup, Docker is used to build container images. Developers create Dockerfiles to define the environment and dependencies needed for the application. These images are then pushed to a container registry (e.g., Docker Hub or a private registry), from where Kubernetes can pull and run them on any node in the cluster.
Here’s a basic workflow for Docker in a Kubernetes environment:
- Create a Dockerfile – Define your application in a
Dockerfile
. - Build the Docker image – Use
docker build
to create an image. - Push the image to a registry – Use
docker push
to upload the image. - Create a Kubernetes deployment – Define a deployment configuration that specifies how many replicas of the container should be running, the resource limits, and environment variables.
- Deploy the application – Kubernetes automatically pulls the image and deploys it across the cluster.
# Example Dockerfile for a simple Node.js app
FROM node:14-alpine
WORKDIR /app
COPY package.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "start"]
Once the Dockerfile is built into an image, you can deploy it to Kubernetes with a deployment YAML:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app
image: myrepo/my-app:latest
ports:
- containerPort: 3000
The Future of Docker in Kubernetes
Looking ahead, Docker's role within Kubernetes will continue to be vital, but Kubernetes itself will evolve further. Features like Helm charts for simplifying deployments, the ability to use multiple container runtimes (e.g., containerd or CRI-O), and better integration with cloud-native technologies like Istio for service mesh management will shape the future of container orchestration.
Moreover, Docker's integration with Kubernetes will become even more streamlined with tools like Docker Desktop for developers and new extensions for Kubernetes that offer enhanced support for container development workflows.
16.2 Edge Computing with Docker
What is Edge Computing?
Edge computing refers to the practice of processing data closer to the location where it is generated rather than sending it to a centralized data center or cloud. This is particularly useful for applications that require low latency or need to operate in environments where internet connectivity is intermittent or unreliable.
Edge computing is gaining traction in industries like IoT (Internet of Things), autonomous vehicles, smart cities, and industrial automation, where large amounts of data are generated at the "edge" (i.e., at the source of data). Instead of transmitting all this data back to a centralized server for processing, edge computing enables local processing to make real-time decisions and reduce reliance on bandwidth.
Docker in Edge Computing
Docker plays a crucial role in enabling edge computing because containers can be deployed on edge devices, providing a lightweight, portable, and consistent runtime for applications. With Docker, developers can package their applications along with the required dependencies into a container, which can then run on various edge devices like IoT sensors, gateways, routers, or even mobile devices.
The main advantage of Docker in edge computing is that it allows you to treat edge devices like mini-clouds. Instead of worrying about the underlying hardware or OS, developers can focus on building containers that will run anywhere.
Use Cases for Docker in Edge Computing
- IoT Devices – In an IoT setup, Docker containers can be deployed on edge devices to process sensor data locally. This reduces the latency of sending data to a central server for processing and provides faster insights.
- Autonomous Vehicles – Containers can run on edge computing platforms inside vehicles, handling tasks like real-time object detection or navigation without needing constant cloud communication.
- Smart Cities – Edge nodes in smart cities, such as traffic lights or surveillance cameras, can use Docker to process data and make real-time decisions, such as rerouting traffic or detecting anomalies.
Deploying Docker Containers to Edge Devices
In an edge environment, managing Docker containers is more complex because you're dealing with many devices that may be located in remote or hard-to-reach locations. Solutions like K3s (a lightweight Kubernetes distribution for edge and IoT) can simplify the management of containerized applications across a fleet of edge devices.
Here’s a simple example of deploying Docker containers to an edge device using docker-compose
, which allows you to manage multi-container applications:
version: '3'
services:
sensor-service:
image: my-sensor-service:latest
environment:
- SENSOR_TYPE=temperature
ports:
- "8080:8080"
analytics-service:
image: my-analytics-service:latest
environment:
- ANALYTICS_MODEL=regression
ports:
- "9090:9090"
Using Docker Compose, you can deploy this multi-service application to edge devices that are running Docker, managing local sensor data and analytics with minimal overhead.
The Future of Docker in Edge Computing
The future of Docker in edge computing lies in its ability to handle distributed applications with minimal resources. As 5G networks roll out, the need for edge computing will increase, and Docker will likely become the de facto solution for managing applications at the edge.
Docker is also expected to play a role in hybrid edge-cloud environments, where applications can run partly on the edge and partly in the cloud. This type of hybrid architecture will allow companies to optimize performance, reduce costs, and ensure the reliability of their applications.
16.3 Serverless and Docker
What is Serverless Computing?
Serverless computing is a cloud-native development model that abstracts the underlying infrastructure, allowing developers to focus purely on their code. In a serverless architecture, developers write functions that are executed in response to events, and the cloud provider automatically handles provisioning, scaling, and resource management.
Serverless computing has grown popular due to its scalability, reduced operational overhead, and pay-per-execution model. Platforms like AWS Lambda, Google Cloud Functions, and Azure Functions allow developers to run code in response to HTTP requests, database changes, or other events, without needing to worry about managing servers or containers.
Docker and Serverless Computing
While serverless computing abstracts infrastructure management away, Docker still plays an important role. Docker can be used to package serverless functions as containers, allowing them to be executed in a serverless environment. The combination of Docker and serverless provides developers with a consistent environment for both local development and production deployment.
Serverless platforms, like AWS Lambda, support Docker containers as execution environments. With AWS Lambda's container image support, you can package your serverless function inside a Docker container, push it to a container registry, and AWS will automatically deploy and manage the execution of that container.
Example: Dockerizing a Serverless Function
Here’s an example of creating a simple serverless application using AWS Lambda and Docker:
- Create a simple Python function that handles an HTTP request:
def lambda_handler(event, context):
return {
'statusCode': 200,
'body': 'Hello from Dockerized Lambda!'
}
- Create a Dockerfile to package this function into a container:
FROM public.ecr.aws/lambda/python:3.8
# Copy the function code into the container
COPY app.py .
# Set the entry point for the Lambda function
CMD ["app.lambda_handler"]
- Build and push the Docker image to AWS ECR (Elastic Container Registry):
docker build -t my-lambda-function .
aws ecr create-repository --repository-name my-lambda-repo
docker tag my-lambda-function:
latest <aws_account_id>.dkr.ecr.<region>.amazonaws.com/my-lambda-repo:latest
docker push <aws_account_id>.dkr.ecr.<region>.amazonaws.com/my-lambda-repo:latest
- Deploy the Lambda function using the AWS Management Console or CLI.
This example shows how Docker can be used to containerize serverless functions, providing a more consistent development and deployment experience across different environments.
The Future of Docker in Serverless
In the future, Docker will likely become an even more integral part of the serverless ecosystem. The flexibility of containers allows serverless platforms to run more complex workloads that may not be possible with traditional serverless models. Docker also provides portability between cloud providers, enabling developers to move serverless workloads between different platforms seamlessly.
As more cloud platforms support containerized serverless functions, Docker will become the preferred method for packaging and deploying serverless applications.
16.4 The Evolution of Containerization
The Early Days of Containers
Containerization began as a way to isolate applications and their dependencies into lightweight, portable units that could run anywhere. Technologies like chroot and FreeBSD jails were precursors to modern containers. However, these early systems lacked the standardization and ease of use that Docker and containers today offer.
When Docker was introduced in 2013, it made containerization accessible to a wider audience by providing an easy-to-use CLI and a registry (Docker Hub) for sharing container images. Docker simplified container creation, management, and deployment, enabling developers to package applications along with all their dependencies into a single unit of execution.
The Emergence of Container Orchestration
As container adoption grew, so did the need for tools to manage large numbers of containers. Kubernetes emerged as the leader in this space, providing automated deployment, scaling, and management of containerized applications.
In addition to Kubernetes, other container orchestration tools like Docker Swarm and Apache Mesos were developed to help developers handle complex containerized applications. However, Kubernetes quickly gained dominance due to its rich feature set, large community support, and its integration with other cloud-native technologies.
The Future of Containers
Looking ahead, containers will continue to evolve. Here are some key trends to watch:
- Multi-cloud and Hybrid Cloud: Containers enable applications to run across different cloud providers and on-premises environments. This will lead to an increased focus on hybrid and multi-cloud strategies, where containers serve as the bridge between diverse infrastructures.
- Container Security: As containers become more pervasive, security will be a critical concern. Technologies like container scanning, runtime protection, and security policy enforcement will continue to evolve.
- Improved Developer Experience: Tools like Docker Compose, Docker Desktop, and Helm charts will further streamline the developer experience, making containerized application development more accessible and efficient.
Conclusion
The future of Docker and containers is bright. As Kubernetes continues to evolve as the container orchestrator of choice, Docker will remain at the core of the containerization ecosystem. Docker will also continue to play a key role in emerging technologies like edge computing, serverless computing, and hybrid cloud architectures, enabling developers to deploy applications in more flexible, efficient, and scalable ways. The continued evolution of containerization will drive innovation in software development, creating new possibilities for applications in the cloud, on the edge, and beyond.
Appendices
17.1 Docker CLI Reference
The Docker CLI (Command Line Interface) is one of the primary ways to interact with Docker, the popular platform for containerization. The Docker CLI provides commands that help users to build, manage, and deploy containers and images. Understanding these commands and their usage is critical for managing containers efficiently, whether you're working locally or in a production environment.
1.1 Basic Structure of Docker Commands
Docker commands are typically structured as follows:
docker [command] [subcommand] [options] [arguments]
docker
: The main CLI tool.[command]
: The primary action to be performed, such asrun
,build
,pull
,push
,exec
,logs
, etc.[subcommand]
: Additional details to specify the action. For example, withdocker build
, you might use--file
to specify a Dockerfile.[options]
: Optional flags that modify the command behavior. For example,-t
for tagging an image.[arguments]
: Values required by the command, like container IDs or image names.
Let's break down a few key Docker commands.
1.2 Core Docker Commands
docker run
The docker run
command is one of the most commonly used commands to start a new container. It runs a container from a specified image.
docker run [OPTIONS] IMAGE [COMMAND] [ARG...]
- OPTIONS: Flags that modify the behavior, such as
-d
(detached mode),-p
(port forwarding), or-e
(environment variables). - IMAGE: The name of the Docker image from which to create the container.
- COMMAND: Optional override of the default command in the Docker image.
- ARG…: Arguments to pass to the command.
Example:
docker run -d -p 8080:80 --name web-server nginx
This command will:
- Start a new container from the
nginx
image. - Run it in detached mode (
-d
). - Forward port 8080 on the host machine to port 80 inside the container (
-p 8080:80
). - Name the container
web-server
.
docker build
The docker build
command is used to create an image from a Dockerfile.
docker build [OPTIONS] PATH | URL | -
- PATH: The path to the directory containing the Dockerfile.
- OPTIONS: Flags like
-t
for tagging the image.
Example:
docker build -t my-app .
This will build a Docker image named my-app
from the Dockerfile in the current directory (.
).
docker ps
The docker ps
command shows a list of all running containers.
docker ps [OPTIONS]
Example:
docker ps -a
This command will show all containers, including the ones that are stopped.
docker exec
The docker exec
command is used to execute commands inside a running container. This is useful for troubleshooting or running commands without modifying the container image.
docker exec [OPTIONS] CONTAINER COMMAND [ARG...]
Example:
docker exec -it web-server bash
This command will start an interactive (-it
) bash shell inside the web-server
container.
docker logs
The docker logs
command allows you to view the logs of a running or stopped container.
docker logs [OPTIONS] CONTAINER
Example:
docker logs web-server
This command will display the logs from the web-server
container.
docker stop and docker start
The docker stop
command stops a running container, while docker start
starts a previously stopped container.
docker stop CONTAINER
docker start CONTAINER
Example:
docker stop web-server
docker start web-server
docker images
The docker images
command lists all available Docker images on the local system.
docker images [OPTIONS]
Example:
docker images
This will display the list of images, including details like the image name, tag, and size.
17.2 Docker Command Cheatsheet
Docker is rich with commands and options. To help you navigate through them quickly, here is a cheatsheet summarizing the most important commands and their use cases.
2.1 Image and Container Management
Command | Description |
---|---|
docker build . |
Build an image from the current directory (Dockerfile) |
docker run [image] |
Run a container from an image |
docker run -d [image] |
Run a container in detached mode |
docker run -p 8080:80 [image] |
Map a port from the container to the host machine |
docker ps |
List running containers |
docker ps -a |
List all containers (running and stopped) |
docker stop [container] |
Stop a running container |
docker start [container] |
Start a stopped container |
docker rm [container] |
Remove a stopped container |
docker rmi [image] |
Remove an image from the local machine |
2.2 Container Interaction
Command | Description |
---|---|
docker exec -it [container] bash |
Execute a bash shell inside a container |
docker exec -it [container] [cmd] |
Execute a specific command in a running container |
docker logs [container] |
View the logs of a container |
docker inspect [container] |
Get detailed information about a container |
docker top [container] |
Display running processes inside a container |
2.3 Image Management
Command | Description |
---|---|
docker images |
List all images available locally |
docker pull [image] |
Pull an image from Docker Hub or another registry |
docker tag [image] [tag] |
Tag an image with a specific tag |
docker push [image] |
Push an image to a Docker registry |
docker build -t [name] [dir] |
Build an image from a directory with a Dockerfile |
docker save [image] > [file] |
Save an image to a tarball file |
2.4 Docker Network Management
Command | Description |
---|---|
docker network ls |
List all Docker networks |
docker network create [name] |
Create a new Docker network |
docker network inspect [name] |
Get detailed information about a specific network |
docker network connect [net] [container] |
Connect a container to a network |
2.5 Docker Volume Management
Command | Description |
---|---|
docker volume ls |
List all Docker volumes |
docker volume create [name] |
Create a new Docker volume |
docker volume inspect [name] |
Get details about a specific Docker volume |
docker volume rm [name] |
Remove a Docker volume |
17.3 Additional Resources
Docker is a vast ecosystem with many tools, concepts, and best practices that can enhance productivity. Below are some key resources for deepening your understanding and keeping up to date with Docker.
3.1 Official Docker Documentation
The official Docker documentation is an invaluable resource for any Docker user, from beginners to experts. It includes comprehensive guides, reference materials, and tutorials.
- Docker Docs: https://docs.docker.com
The documentation is organized by topics such as:
- Getting started with Docker
- Container concepts
- Docker Compose for multi-container applications
- Docker Swarm for orchestration
- Best practices for Docker images and containers
3.2 Docker GitHub Repository
For users who want to dive into the source code or contribute to Docker’s development, the Docker GitHub repository is the place to go.
- Docker GitHub: https://github.com/docker
The repository contains:
- Docker Engine (the core of Docker)
- Docker Compose (tool for defining multi-container applications)
- Docker Desktop (for Mac and Windows users)
- Other official projects like Docker CLI, Docker Hub, and Docker Compose
3.3 Docker Training and Certifications
Docker offers various training resources to help you become proficient with the platform. If you're looking for structured learning or certification, Docker provides official training and certification programs.
- Docker Training: https://www.docker.com/training
- Docker Certification: [https://www.docker.com/certification](https://www.d
ocker.com/certification)
The Docker Certified Associate (DCA) is a professional certification designed for developers, system admins, and DevOps engineers who want to demonstrate their expertise in Docker.
3.4 Community Forums and Support
Joining the Docker community can be a great way to learn from others, ask questions, and stay up to date with the latest developments. Below are some active forums and platforms where you can engage with other Docker users:
- Docker Community Forums: https://forums.docker.com
- Stack Overflow: Many Docker-related questions can be found and answered here. The Docker tag on Stack Overflow is widely used.
- Docker Slack: Docker has an active Slack community where users share tips and discuss best practices.
3.5 Blogs and Tutorials
There are many independent blogs and websites where Docker professionals share tutorials, guides, and best practices. Here are a few notable ones:
- Docker Blog: https://www.docker.com/blog
- TutorialsPoint (Docker Tutorial): https://www.tutorialspoint.com/docker/index.htm
- Dev.to Docker Articles: https://dev.to/t/docker
- DigitalOcean Docker Tutorials: https://www.digitalocean.com/community/tags/docker
3.6 Books
If you're looking for books to deepen your Docker knowledge, here are some well-reviewed titles:
- "Docker Deep Dive" by Nigel Poulton: A comprehensive guide that explains Docker concepts, CLI commands, and usage in detail.
- "The Docker Book" by James Turnbull: A great book for beginners, providing hands-on tutorials and examples of Docker commands and workflows.
- "Docker for Developers" by Richard Bullington-McGuire: Focuses on how Docker benefits developers, including examples of using Docker in a CI/CD pipeline.
Conclusion
Docker is an indispensable tool for modern development and DevOps workflows. Mastering the Docker CLI, knowing essential commands, and staying informed with the latest updates are crucial for any professional using Docker regularly. By leveraging the Docker CLI reference, cheatsheet, and additional resources, you can navigate through Docker’s vast ecosystem and increase your productivity and containerization expertise.