Understanding Garbage Collection: A Boon for Developers, But Not Without Trade-offs
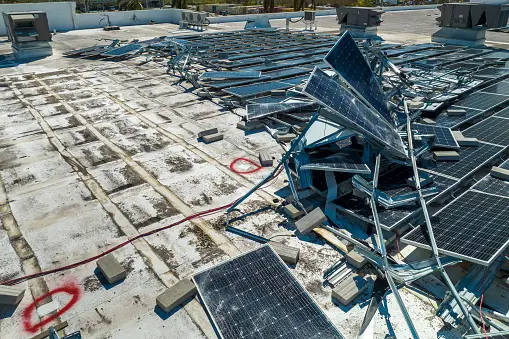
Memory management is vital to the dynamic world of programming in order to guarantee effective application execution. Garbage collection (GC) is a popular method of managing memory. This essay dives into the realm of GC, examining its benefits, drawbacks, and implementation differences amongst well-known programming languages.
What is Garbage Collection?
Garbage collection (GC) is an automated memory management technique employed by certain programming languages. It alleviates developers from the burden of manually allocating and deallocating memory for objects during program execution. GC automatically identifies and reclaims memory occupied by unused objects, preventing memory leaks and simplifying development.
The Garbage Collection Cycle:
- Object Allocation: When a program creates an object, memory is allocated on the heap (a dedicated memory region for object storage).
- Object Usage: As the program executes, objects are referenced and utilized within the code.
- Object Inaccessibility: Over time, objects may become unreachable or “garbage” – no longer referenced by any part of the program.
- Garbage Collection: The GC kicks in, employing various algorithms to identify these unreachable objects.
- Memory Reclamation: Once identified, the memory occupied by garbage objects is reclaimed and made available for future allocations.
Benefits of Garbage Collection:
- Reduced Development Complexity: GC eliminates the need for manual memory management, making development faster and less error-prone. Developers can focus on core functionalities without worrying about memory leaks or deallocation.
- Improved Memory Efficiency: GC prevents memory leaks, a common issue in manual memory management, where unused memory remains allocated. This ensures efficient memory utilization throughout program execution.
- Safer Programming Practices: GC safeguards against memory-related errors such as dangling pointers (pointers referencing deallocated memory). This leads to more robust and reliable programs.
- Simplified Debugging: By handling memory management, GC reduces the debugging burden associated with memory leaks and other memory-related issues. Developers can focus on identifying logical errors within their code.
Drawbacks of Garbage Collection:
- Performance Overhead: GC introduces background processes to analyze memory usage and reclaim unused space. This can cause occasional pauses in program execution, potentially impacting performance in real-time systems.
- Reduced Predictability: Manual memory management allows for precise control over memory allocation and deallocation. With GC, the timing of memory reclamation is less predictable, making performance optimization more challenging.
- Memory Fragmentation: As GC frees memory chunks, it can leave scattered unused gaps in memory. This fragmentation can reduce efficiency as the program searches for contiguous free space for new allocations.
- Limited Control: Developers have less control over memory usage with GC. This can make it difficult to optimize memory usage for specific scenarios or identify memory leaks.
- Not ideal for Resource-Constrained Systems: GC might not be suitable for systems with limited memory or processing power. The overhead of GC can be significant on such systems.
Garbage Collection in Action: Language-Specific Implementations
While the core concept of GC remains consistent, its implementation details vary across programming languages. Here’s a glimpse into how GC works in some popular languages:
Java:
- Java employs a generational garbage collector. It divides the heap into young and old generations. Younger objects are more likely to become garbage, while older objects tend to have a longer lifespan.
- The young generation undergoes frequent collections using a copying algorithm. Objects that survive multiple collections are promoted to the older generation.
- The older generation undergoes less frequent but more complex collections using mark-and-sweep algorithms.
C#:
- C# utilizes a generational garbage collector similar to Java’s. It combines copying and mark-and-sweep algorithms for efficient memory management.
- The .NET runtime, on which C# applications run, manages the garbage collector. Developers can access some GC functionalities but generally don’t need to directly manage memory allocation or deallocation.
Python:
- Python has a simple and efficient automatic garbage collector employing reference counting. Each object maintains a reference count, tracking the number of references pointing to it.
- When the reference count reaches zero, signifying no references remaining, the object is considered garbage and gets reclaimed by the GC.
- Python’s GC is well-suited for general-purpose applications but might not be ideal for performance-critical scenarios.
C++:
- C++ stands out as it doesn’t have built-in garbage collection. Memory management in C++ is manual, requiring developers to allocate and deallocate memory using operators like new and delete.
- While offering fine-grained control, manual memory management can be error-prone and lead to memory leaks if not handled carefully. Smart pointers and RAII (Resource Acquisition Is Initialization) techniques can help mitigate these risks.
Choosing the Right Approach:
The decision of whether to use garbage collection or manual memory management depends on various factors like project requirements, performance needs, and developer expertise. Here’s a breakdown to guide your choice:
When to Choose Garbage Collection:
- Rapid Development: GC simplifies development by automating memory management, making it ideal for projects with tight deadlines or rapid prototyping needs.
- Reduced Development Errors: GC safeguards against memory-related errors, leading to more robust and reliable programs, especially beneficial for complex applications.
- Focus on Core Logic: Developers can concentrate on core functionalities without being bogged down by manual memory management, improving overall development efficiency.
- General-Purpose Applications: GC is well-suited for applications where real-time performance isn’t a paramount concern, such as web development, data analysis, and enterprise software.
When to Consider Manual Memory Management:
- Performance-Critical Systems: In real-time systems or applications demanding absolute control over memory usage, manual memory management offers predictability and avoids potential GC pauses.
- Resource-Constrained Environments: For systems with limited memory or processing power, the overhead of GC can be significant. Manual memory management allows for fine-grained control and optimization.
- Embedded Systems: Memory management in embedded systems often requires specific control over memory allocation and deallocation due to resource limitations.
- Experienced Developers: Manual memory management necessitates a thorough understanding of memory allocation and deallocation principles. It’s best suited for projects with experienced developers who can effectively manage memory usage.
Hybrid Approaches:
Some programming languages, like C++, allow for a hybrid approach. While primarily relying on manual memory management, developers can leverage smart pointers that automate memory deallocation when the pointer goes out of scope. This offers a balance between control and convenience.
Advanced Garbage Collection Topics:
Beyond the fundamentals, garbage collection encompasses various advanced concepts and techniques:
- Generational Garbage Collection: As discussed earlier, dividing the heap into generations with different collection frequencies optimizes performance based on object lifespans.
- Compacting Garbage Collection: This approach rearranges objects in memory to eliminate fragmentation and improve memory utilization.
- Concurrent Garbage Collection: GC can run concurrently with program execution, minimizing pauses and improving responsiveness in real-time systems.
- Deterministic Garbage Collection: This technique aims for predictable GC pauses, making it suitable for real-time systems with strict timing constraints.
The Future of Garbage Collection:
Research in garbage collection is ongoing, with a focus on improving efficiency, reducing overhead, and adapting to emerging programming paradigms like concurrent programming and big data applications. Here are some potential future directions:
- Improved Algorithms: Development of more efficient garbage collection algorithms that minimize pauses and optimize memory usage.
- Language-Specific Optimizations: Tailoring GC techniques to specific language features and runtime environments for better performance.
- Real-Time GC: Advancements in real-time garbage collection to ensure predictable pauses and meet the stringent timing requirements of real-time systems.
- Memory-Aware Programming: Languages and tools that provide developers with better insights into memory usage and potential optimizations alongside GC.
Conclusion:
Reducing the likelihood of memory-related errors and streamlining development are two benefits of garbage collection as a memory management tool. But there is not a single solution that works for everyone. Making educated decisions requires an understanding of the benefits, drawbacks, and language-specific implementations. Developers are able to construct reliable and effective applications by selecting the best memory management strategy by carefully analysing project requirements and performance requirements.